用C++写一个学校人力资源管理系统,并且可以输入信息
时间: 2024-03-02 08:49:56 浏览: 21
好的,学校人力资源管理系统是一个比较复杂的系统,需要考虑很多方面的因素,比如员工的基本信息、考勤记录、工资发放、培训计划等等。以下是一个简单的C++代码框架,您可以根据需要进行修改和完善。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 定义员工类
class Employee {
public:
Employee(string name, string gender, int age, string department, string position, int salary) :
name(name), gender(gender), age(age), department(department), position(position), salary(salary) {}
string getName() { return name; }
string getGender() { return gender; }
int getAge() { return age; }
string getDepartment() { return department; }
string getPosition() { return position; }
int getSalary() { return salary; }
private:
string name; // 姓名
string gender; // 性别
int age; // 年龄
string department; // 部门
string position; // 职位
int salary; // 工资
};
// 定义员工管理类
class EmployeeManager {
public:
void addEmployee(Employee employee) { employees.push_back(employee); } // 添加员工
void removeEmployee(int index) { employees.erase(employees.begin() + index); } // 删除员工
void printAllEmployees() { // 打印所有员工的信息
for (int i = 0; i < employees.size(); i++) {
cout << "姓名:" << employees[i].getName() << endl;
cout << "性别:" << employees[i].getGender() << endl;
cout << "年龄:" << employees[i].getAge() << endl;
cout << "部门:" << employees[i].getDepartment() << endl;
cout << "职位:" << employees[i].getPosition() << endl;
cout << "工资:" << employees[i].getSalary() << endl;
cout << endl;
}
}
private:
vector<Employee> employees; // 员工列表
};
int main() {
EmployeeManager manager; // 员工管理器
// TODO: 添加员工,删除员工,打印员工信息等操作
return 0;
}
```
您可以在main函数中进行添加员工、删除员工、打印员工信息等操作。比如,添加一个员工的方式如下:
```c++
Employee employee("张三", "男", 25, "人事部", "人事专员", 5000);
manager.addEmployee(employee);
```
当然,这只是一个简单的框架,您可以根据实际需求进行修改和完善。
相关推荐
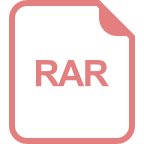
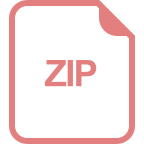














