用C++写一个学校人力资源管理系统,并且可以添加员工信息和删除员工信息,不使用auto函数
时间: 2024-03-09 17:45:57 浏览: 18
好的,以下是一个简单的学校人力资源管理系统的C++代码,它可以添加和删除员工信息,不使用auto函数:
```
#include <iostream>
#include <cstring>
using namespace std;
// 定义员工结构体
struct Employee {
string name; // 姓名
int age; // 年龄
string position; // 职位
};
// 添加员工信息
void addEmployee(Employee *employees, int &count) {
cout << "请输入员工姓名:";
cin >> employees[count].name;
cout << "请输入员工年龄:";
cin >> employees[count].age;
cout << "请输入员工职位:";
cin >> employees[count].position;
count++;
cout << "添加成功!" << endl;
}
// 删除员工信息
void deleteEmployee(Employee *employees, int &count) {
string name; // 要删除的员工姓名
int index = -1; // 要删除的员工在数组中的下标
cout << "请输入要删除的员工姓名:";
cin >> name;
for (int i = 0; i < count; i++) {
if (employees[i].name == name) {
index = i;
break;
}
}
if (index == -1) {
cout << "没有找到该员工信息!" << endl;
} else {
for (int i = index; i < count - 1; i++) {
employees[i] = employees[i + 1];
}
count--;
cout << "删除成功!" << endl;
}
}
// 显示员工信息
void showEmployees(Employee *employees, int count) {
cout << "员工姓名\t员工年龄\t员工职位" << endl;
for (int i = 0; i < count; i++) {
cout << employees[i].name << "\t\t" << employees[i].age << "\t\t" << employees[i].position << endl;
}
}
int main() {
const int MAX_EMPLOYEES = 100; // 最大员工数
Employee employees[MAX_EMPLOYEES]; // 员工数组
int count = 0; // 员工数
while (true) {
cout << "请选择要执行的操作:" << endl;
cout << "1. 添加员工信息" << endl;
cout << "2. 删除员工信息" << endl;
cout << "3. 显示员工信息" << endl;
cout << "4. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addEmployee(employees, count);
break;
case 2:
deleteEmployee(employees, count);
break;
case 3:
showEmployees(employees, count);
break;
case 4:
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
}
}
return 0;
}
```
在这个程序中,我们定义了一个员工结构体,包括员工的姓名、年龄和职位。然后,我们通过实现`addEmployee`、`deleteEmployee`和`showEmployees`函数来添加、删除和显示员工信息。在`main`函数中,我们使用一个无限循环来等待用户的输入,根据用户的选择执行相应的操作,直到用户选择退出程序为止。
相关推荐
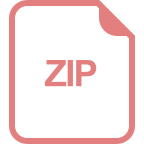
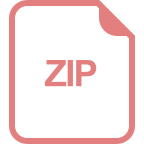
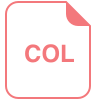
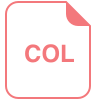
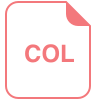
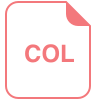
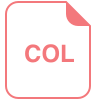









