如何用Java 写生成二维码项目
时间: 2024-01-01 12:04:11 浏览: 33
要使用Java生成二维码,可以使用ZXing库(也称为“zebra crossing”)。以下是在Java中使用ZXing库生成二维码的步骤:
1. 首先,需要下载ZXing库并将其添加到Java项目中。可以从https://github.com/zxing/zxing/downloads下载最新版本。
2. 创建一个Java类,导入必要的库。
```java
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
```
3. 编写一个方法来生成二维码。该方法应该接受一个字符串参数,该参数是要被编码为二维码的内容。该方法应该返回一个BufferedImage对象,该对象包含二维码图像。
```java
public static BufferedImage generateQRCodeImage(String text) throws WriterException, IOException {
QRCodeWriter qrCodeWriter = new QRCodeWriter();
Map<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");
BitMatrix bitMatrix = qrCodeWriter.encode(text, BarcodeFormat.QR_CODE, 200, 200, hints);
BufferedImage bufferedImage = new BufferedImage(200, 200, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < 200; x++) {
for (int y = 0; y < 200; y++) {
bufferedImage.setRGB(x, y, bitMatrix.get(x, y) ? Color.BLACK.getRGB() : Color.WHITE.getRGB());
}
}
return bufferedImage;
}
```
4. 最后,将生成的二维码保存到文件中。可以使用以下代码将图像保存为PNG文件。
```java
BufferedImage qrCodeImage = generateQRCodeImage("https://www.example.com");
File outputFile = new File("qrcode.png");
ImageIO.write(qrCodeImage, "png", outputFile);
```
以上就是使用Java生成二维码的基本步骤。当然,你可以根据实际需求进行更改和扩展。
相关推荐
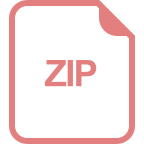
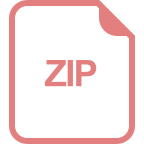














