用python写一个TIC归一化的代码
时间: 2023-12-17 10:11:50 浏览: 32
以下是一个简单的TIC归一化的Python代码,假设有一个质谱数据矩阵`data`,每一行代表一个样本,每一列代表一个离子峰:
``` python
import numpy as np
# 计算每个样本的TIC
tic = np.sum(data, axis=1)
# 计算TIC的平均值
tic_mean = np.mean(tic)
# 计算TIC的标准差
tic_std = np.std(tic)
# 对每个样本进行TIC归一化
data_norm = (data - np.mean(data, axis=0)) / np.std(data, axis=0) * tic_std + tic_mean
```
在这个代码中,首先计算每个样本的TIC,然后计算TIC的平均值和标准差,最后将每个样本的质谱信号进行归一化。归一化的方法是将每个样本的数据减去均值除以标准差,然后乘以TIC的标准差并加上TIC的平均值。这样做可以保证每个样本的TIC都相同,同时保留了每个离子峰的相对强度差异。
相关问题
假定一个数据集,用python写一个TIC归一化代码
可以使用以下Python代码实现TIC归一化:
``` python
import numpy as np
def tic_normalization(data):
# 计算每个样本的TIC(Total Ion Current)
tic = np.sum(data, axis=1)
# 归一化
normalized_data = data / tic[:, np.newaxis]
return normalized_data
```
其中,`data`是一个二维数组,每一行代表一个样本,每一列代表一个特征。函数中,首先计算每个样本的TIC,然后将每个元素除以其对应的TIC,得到归一化后的数据。最后返回归一化后的数据。
用python写一个ai井字棋游戏的代码
好的,以下是一个简单的 Python AI 井字棋游戏的代码:
```python
import random
def draw_board(board):
# This function prints out the board that it was passed.
# "board" is a list of 10 strings representing the board (ignore index 0)
print(' | |')
print(' ' + board[7] + ' | ' + board[8] + ' | ' + board[9])
print(' | |')
print('-----------')
print(' | |')
print(' ' + board[4] + ' | ' + board[5] + ' | ' + board[6])
print(' | |')
print('-----------')
print(' | |')
print(' ' + board[1] + ' | ' + board[2] + ' | ' + board[3])
print(' | |')
def input_player_letter():
# Lets the player type which letter they want to be.
# Returns a list with the player's letter as the first item, and the AI's letter as the second.
letter = ''
while not (letter == 'X' or letter == 'O'):
print('Do you want to be X or O?')
letter = input().upper()
# the first element in the tuple is the player's letter, the second is the AI's letter.
if letter == 'X':
return ['X', 'O']
else:
return ['O', 'X']
def who_goes_first():
# Randomly choose the player who goes first.
if random.randint(0, 1) == 0:
return 'AI'
else:
return 'player'
def make_move(board, letter, move):
board[move] = letter
def is_winner(bo, le):
# Given a board and a player's letter, this function returns True if that player has won.
# We use bo instead of board and le instead of letter so we don't have to type as much.
return ((bo[7] == le and bo[8] == le and bo[9] == le) or # across the top
(bo[4] == le and bo[5] == le and bo[6] == le) or # across the middle
(bo[1] == le and bo[2] == le and bo[3] == le) or # across the bottom
(bo[7] == le and bo[4] == le and bo[1] == le) or # down the left side
(bo[8] == le and bo[5] == le and bo[2] == le) or # down the middle
(bo[9] == le and bo[6] == le and bo[3] == le) or # down the right side
(bo[7] == le and bo[5] == le and bo[3] == le) or # diagonal
(bo[9] == le and bo[5] == le and bo[1] == le)) # diagonal
def get_board_copy(board):
# Make a duplicate of the board list and return it the duplicate.
dupe_board = []
for i in board:
dupe_board.append(i)
return dupe_board
def is_space_free(board, move):
# Return true if the passed move is free on the passed board.
return board[move] == ' '
def get_player_move(board):
# Let the player type in their move.
move = ' '
while move not in '1 2 3 4 5 6 7 8 9'.split() or not is_space_free(board, int(move)):
print('What is your next move? (1-9)')
move = input()
return int(move)
def choose_random_move_from_list(board, movesList):
# Returns a valid move from the passed list on the passed board.
# Returns None if there is no valid move.
possibleMoves = []
for i in movesList:
if is_space_free(board, i):
possibleMoves.append(i)
if len(possibleMoves) != 0:
return random.choice(possibleMoves)
else:
return None
def get_ai_move(board, ai_letter):
# Given a board and the computer's letter, determine where to move and return that move.
if ai_letter == 'X':
player_letter = 'O'
else:
player_letter = 'X'
# Here is our algorithm for our Tic Tac Toe AI:
# First, check if we can win in the next move
for i in range(1, 10):
copy = get_board_copy(board)
if is_space_free(copy, i):
make_move(copy, ai_letter, i)
if is_winner(copy, ai_letter):
return i
# Check if the player could win on their next move, and block them.
for i in range(1, 10):
copy = get_board_copy(board)
if is_space_free(copy, i):
make_move(copy, player_letter, i)
if is_winner(copy, player_letter):
return i
# Try to take one of the corners, if they are free.
move = choose_random_move_from_list(board, [1, 3, 7, 9])
if move != None:
return move
# Try to take the center, if it is free.
if is_space_free(board, 5):
return 5
# Move on one of the sides.
return choose_random_move_from_list(board, [2, 4, 6, 8])
def is_board_full(board):
# Return True if every space on the board has been taken. Otherwise return False.
for i in range(1, 10):
if is_space_free(board, i):
return False
return True
print('Welcome to AI Tic Tac Toe!')
while True:
# Reset the board
the_board = [' '] * 10
player_letter, ai_letter = input_player_letter()
turn = who_goes_first()
print('The ' + turn + ' will go first.')
game_is_playing = True
while game_is_playing:
if turn == 'player':
# Player's turn.
draw_board(the_board)
move = get_player_move(the_board)
make_move(the_board, player_letter, move)
if is_winner(the_board, player_letter):
draw_board(the_board)
print('Hooray! You have won the game!')
game_is_playing = False
else:
if is_board_full(the_board):
draw_board(the_board)
print('The game is a tie!')
break
else:
turn = 'AI'
else:
# AI's turn.
move = get_ai_move(the_board, ai_letter)
make_move(the_board, ai_letter, move)
if is_winner(the_board, ai_letter):
draw_board(the_board)
print('The AI has beaten you! You lose.')
game_is_playing = False
else:
if is_board_full(the_board):
draw_board(the_board)
print('The game is a tie!')
break
else:
turn = 'player'
print('Do you want to play again? (yes or no)')
if not input().lower().startswith('y'):
break
```
希望这个代码能够帮到你!
相关推荐
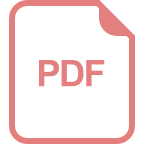
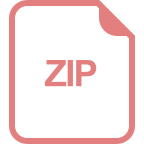












