给入两个ORBSLAM运动轨迹txt,使轨迹对齐代码C++
时间: 2023-07-11 19:05:53 浏览: 154
对于给定的两个ORBSLAM运动轨迹txt文件,可以将它们读入到程序中,并使用ICP(Iterative Closest Point)算法对它们进行对齐。以下是一个简单的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <pcl/point_types.h>
#include <pcl/registration/icp.h>
#include <pcl/io/pcd_io.h>
using namespace std;
int main(int argc, char** argv)
{
// 读入两个轨迹文件
ifstream file1(argv[1]);
ifstream file2(argv[2]);
vector<pcl::PointXYZ> cloud1, cloud2;
// 从第一个文件读入点云
while (!file1.eof())
{
double x, y, z;
file1 >> x >> y >> z;
cloud1.push_back(pcl::PointXYZ(x, y, z));
}
// 从第二个文件读入点云
while (!file2.eof())
{
double x, y, z;
file2 >> x >> y >> z;
cloud2.push_back(pcl::PointXYZ(x, y, z));
}
// 创建一个ICP对象
pcl::IterativeClosestPoint<pcl::PointXYZ, pcl::PointXYZ> icp;
// 设置ICP参数
icp.setInputSource(pcl::PointCloud<pcl::PointXYZ>::Ptr(new pcl::PointCloud<pcl::PointXYZ>(cloud1.begin(), cloud1.end())));
icp.setInputTarget(pcl::PointCloud<pcl::PointXYZ>::Ptr(new pcl::PointCloud<pcl::PointXYZ>(cloud2.begin(), cloud2.end())));
icp.setMaximumIterations(100);
icp.setTransformationEpsilon(1e-8);
// 进行配准
pcl::PointCloud<pcl::PointXYZ> aligned_cloud;
icp.align(aligned_cloud);
// 输出配准结果
cout << "ICP converged with score: " << icp.getFitnessScore() << endl;
cout << "Transformation matrix: " << endl << icp.getFinalTransformation() << endl;
// 将结果保存到PCD文件中
pcl::io::savePCDFile("aligned_cloud.pcd", aligned_cloud);
return 0;
}
```
以上代码使用了PCL库来进行ICP算法的实现。首先,我们从给定的两个轨迹文件中读入点云数据,然后创建一个ICP对象,并设置它的参数。接下来,我们调用`align()`函数进行配准。最后,我们输出配准结果并将结果保存到PCD文件中。
请注意,以上代码仅供参考,并不保证在所有情况下都能正常工作。在实际应用中,您可能需要根据具体情况对代码进行修改和调整。
阅读全文
相关推荐
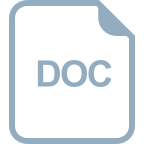
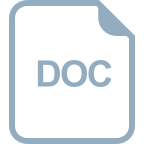
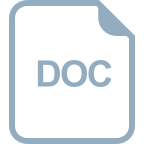



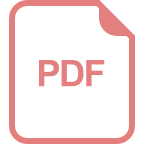
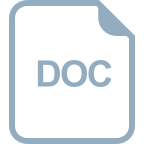
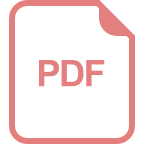
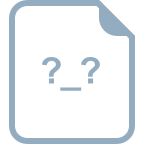
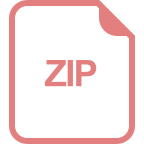
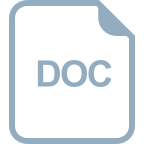
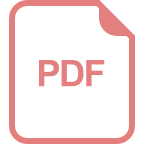
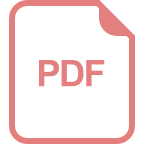
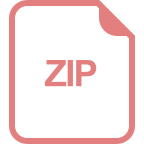