给我一个简单的例子,让我学会使用Python装饰器
时间: 2023-06-10 14:06:17 浏览: 50
好的,以下是一个简单的例子:
```python
def my_decorator(func):
def wrapper():
print("Before the function is called.")
func()
print("After the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
# Call the decorated function
say_hello()
```
以上代码定义了一个装饰器函数`my_decorator`,它一个函数作为参数并返回另一个函数(`wrapper`)。每次调用被装饰的函数时,`my_decorator`都会在函数之前和之后输出内容。
接下来,在`say_hello()`函数定义上面加上`@my_decorator`这个标注,这意味着这个函数被`my_decorator`装饰。最后调用`say_hello()`函数,会输出以下内容:
```
Before the function is called.
Hello!
After the function is called.
```
这就是一个简单的Python装饰器的例子,希望可以帮助你理解装饰器的用法。
相关问题
给我一个稍微复杂的例子,让我学会使用Python装饰器
好的,我可以给您一个稍微复杂的例子来帮助您学习使用Python装饰器。假设您正在编写一个Web应用程序,并且需要在应用程序中对一些敏感函数进行认证。您可以编写一个authentication装饰器来实现这一点。
以下是一个使用authentication装饰器的示例代码:
```
def authentication(func):
def wrapper(*args, **kwargs):
# 在这里实现认证逻辑
# 如果认证成功,则调用原始函数
# 如果认证失败,则返回错误信息
return func(*args, **kwargs)
return wrapper
@authentication
def sensitive_function():
# 在这里实现敏感操作
# 如果认证失败,则敏感操作不会执行
pass
```
在上面的代码中,authentication装饰器接受一个函数作为参数,并返回一个新的函数wrapper。在wrapper函数中,您可以实现认证逻辑,例如检查用户是否已登录或是否具有足够的权限等。如果认证成功,则调用传递给decorator的原始函数(即sensitive_function)。如果认证失败,则返回一个错误消息或者什么都不做。通过将authentication装饰器应用于您的敏感函数,您可以确保只有经过验证的用户才能访问这些函数,从而增加了应用程序的安全性。
给我一个简单的输入密码,判断是否是用户的例子,让我学会使用Python装饰器
好的,关于密码输入的判断,可以使用以下的Python装饰器实现:
```
def check_password(func):
def wrapper(password):
# 检查密码是否符合要求
if len(password) < 6:
print("密码长度不能少于6位!")
return False
# 这里可以添加更多检查密码的规则
# 如果密码符合要求,则调用原函数并返回结果
return func(password)
return wrapper
@check_password
def is_user_password(password):
user_passwords = ['password', '123456', 'qwerty']
if password in user_passwords:
print("密码是用户的例子")
return True
else:
print("密码不是用户的例子")
return False
# 测试装饰器
is_user_password('password')
is_user_password('123')
```
这里定义了一个`check_password`装饰器,并在内部定义了一个`wrapper`函数。`wrapper`函数会检查输入的密码是否符合要求,并根据结果决定是否调用原函数。在`is_user_password`函数上添加了装饰器`@check_password`,即可实现检查密码的功能。
第一个测试输出是"密码是用户的例子",因为'password'在用户密码列表中。第二个测试输出是"密码长度不能少于6位!",因为长度不符合要求。
相关推荐
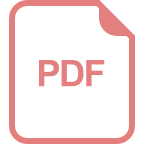
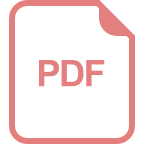
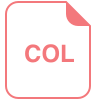
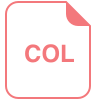
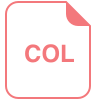









