写一个基于opencv与pyqt的火焰识别程序
时间: 2023-12-10 21:03:35 浏览: 111
好的,这是一个基于OpenCV和PyQt的火焰识别程序的示例代码。在此示例代码中,我们将从摄像头中读取视频,并在PyQt界面上显示检测到的火焰区域。
```
import sys
import cv2
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout
from PyQt5.QtCore import QTimer
class FlameDetector(QWidget):
def __init__(self):
super().__init__()
# 创建界面元素
self.label = QLabel(self)
self.layout = QVBoxLayout()
self.layout.addWidget(self.label)
self.setLayout(self.layout)
# 创建定时器
self.timer = QTimer(self)
self.timer.timeout.connect(self.detect_flame)
self.timer.start(30)
# 打开摄像头
self.cap = cv2.VideoCapture(0)
def detect_flame(self):
# 读取帧
ret, frame = self.cap.read()
# 进行火焰检测
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (11, 11), 0)
edged = cv2.Canny(blurred, 50, 150)
(cnts, _) = cv2.findContours(edged.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for c in cnts:
area = cv2.contourArea(c)
if area > 500:
x,y,w,h = cv2.boundingRect(c)
cv2.rectangle(frame,(x,y),(x+w,y+h),(0,0,255),2)
# 将帧转换为PyQt格式并显示在界面上
pixmap = QPixmap.fromImage(QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888).rgbSwapped())
self.label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication(sys.argv)
detector = FlameDetector()
detector.show()
sys.exit(app.exec_())
```
在此示例代码中,我们创建了一个名为FlameDetector的QWidget子类,该子类包含一个QLabel用于显示视频帧。我们还创建了一个定时器,该定时器每隔30毫秒调用detect_flame方法,以便在每个时间间隔内检测视频帧中的火焰。在detect_flame方法中,我们使用OpenCV的火焰检测算法检测视频帧中的火焰,并将检测到的火焰区域绘制在帧上。然后,我们将帧转换为PyQt格式并在QLabel中显示它。
要运行此示例代码,您可以将其保存为flame_detector.py文件,并在命令行中运行以下命令:
```
python flame_detector.py
```
然后,您将看到一个PyQt窗口,在该窗口中,您将看到从摄像头中读取的视频,并且所有检测到的火焰区域都将用红色矩形框出。
阅读全文
相关推荐

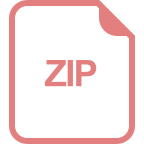
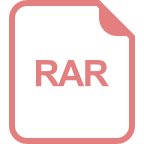
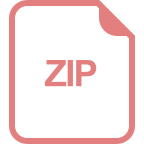
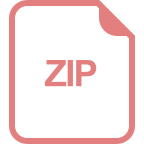
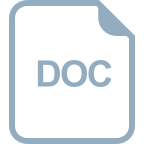
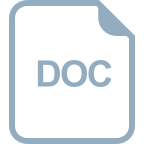


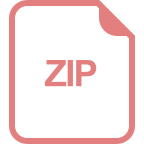
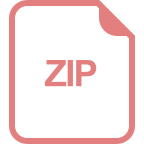