python编程返回每个学生的学号和最高分
时间: 2023-05-30 10:02:46 浏览: 199
这个问题需要具体的数据结构和数据输入方式才能给出代码实现。以下是一种可能的实现方式:
假设学生的信息以字典方式存储,键为学号,值为包含学生姓名和成绩的字典,如下所示:
```
students = {
'001': {'name': 'Alice', 'score': 85},
'002': {'name': 'Bob', 'score': 92},
'003': {'name': 'Charlie', 'score': 78},
'004': {'name': 'David', 'score': 90},
'005': {'name': 'Emily', 'score': 86}
}
```
可以编写一个函数,输入为学生信息的字典,输出为包含每个学生的学号和最高分的列表,如下所示:
```python
def get_highest_scores(students):
highest_scores = []
for id, info in students.items():
highest_score = max(info['score'] for id, info in students.items())
if info['score'] == highest_score:
highest_scores.append({'id': id, 'score': highest_score})
return highest_scores
```
该函数使用了一个列表来保存每个学生的学号和最高分,对每个学生的成绩进行一次最大值计算,如果该学生的成绩等于最大值,则将该学生的学号和最高分添加到列表中。最后返回列表。
示例运行代码如下:
```python
students = {
'001': {'name': 'Alice', 'score': 85},
'002': {'name': 'Bob', 'score': 92},
'003': {'name': 'Charlie', 'score': 78},
'004': {'name': 'David', 'score': 90},
'005': {'name': 'Emily', 'score': 86}
}
highest_scores = get_highest_scores(students)
print(highest_scores)
```
输出结果为:
```
[{'id': '002', 'score': 92}]
```
表示学号为002的学生获得了最高分92分。
相关推荐









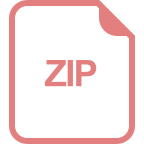
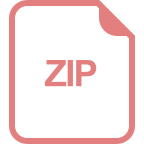
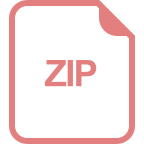
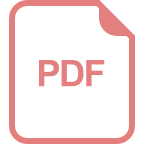