vue 父子组件值双向绑定
时间: 2023-08-29 15:07:13 浏览: 94
在 Vue 中,可以使用 `v-bind` 和 `v-on` 指令实现父子组件值的双向绑定。
首先,在父组件中使用 `v-bind` 绑定一个变量到子组件的 `prop` 上,例如:
```html
<template>
<div>
<child-component v-bind:childValue="parentValue"></child-component>
</div>
</template>
<script>
export default {
data() {
return {
parentValue: ''
}
}
}
</script>
```
然后,在子组件中使用 `props` 接收父组件传递的值,并使用 `v-model` 绑定子组件内部的变量,例如:
```html
<template>
<div>
<input v-model="childValue">
</div>
</template>
<script>
export default {
props: {
childValue: {
type: String,
default: ''
}
}
}
</script>
```
这样,当子组件内部的 `input` 元素发生变化时,父组件的 `parentValue` 也会跟着改变。
注意,如果子组件需要修改父组件传递的值,需要使用 `$emit` 方法触发一个自定义事件,例如:
```html
<template>
<div>
<input v-model="childValue" @input="handleChange">
</div>
</template>
<script>
export default {
props: {
childValue: {
type: String,
default: ''
}
},
methods: {
handleChange() {
this.$emit('update:childValue', this.childValue)
}
}
}
</script>
```
在父组件中,可以使用 `v-on` 监听子组件触发的自定义事件,并更新父组件的变量,例如:
```html
<template>
<div>
<child-component v-bind:childValue="parentValue" v-on:update:childValue="handleChildValueChange"></child-component>
</div>
</template>
<script>
export default {
data() {
return {
parentValue: ''
}
},
methods: {
handleChildValueChange(value) {
this.parentValue = value
}
}
}
</script>
```
这样,当子组件内部的 `input` 元素发生变化时,不仅父组件的 `parentValue` 会跟着改变,子组件也可以修改父组件的值。
阅读全文
相关推荐
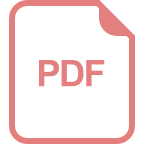
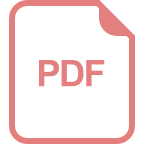
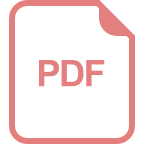

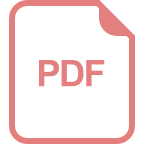








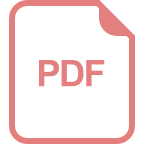
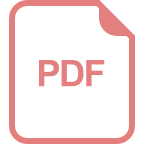
