用Java帮我写一个贪吃蛇
时间: 2024-01-06 13:03:56 浏览: 70
好的,我可以帮你写一个简单的贪吃蛇游戏。下面是代码实现:
```java
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.LinkedList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Snake extends JFrame {
private static final long serialVersionUID = 1L;
private static final int ROWS = 20;
private static final int COLS = 20;
private static final int BLOCK_SIZE = 20;
private JPanel contentPane;
private LinkedList<Point> snake;
private Point food;
private boolean isRunning;
private boolean isPaused;
private int score;
private int direction;
private Random rand;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Snake frame = new Snake();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public Snake() {
setTitle("Snake");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, COLS * BLOCK_SIZE + 6, ROWS * BLOCK_SIZE + 29);
setLocationRelativeTo(null);
contentPane = new JPanel();
setContentPane(contentPane);
contentPane.setLayout(null);
addKeyListener(new KeyListener() {
public void keyTyped(KeyEvent e) {}
public void keyReleased(KeyEvent e) {}
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
if (direction != 2) direction = 0;
break;
case KeyEvent.VK_RIGHT:
if (direction != 3) direction = 1;
break;
case KeyEvent.VK_DOWN:
if (direction != 0) direction = 2;
break;
case KeyEvent.VK_LEFT:
if (direction != 1) direction = 3;
break;
case KeyEvent.VK_SPACE:
isPaused = !isPaused;
break;
}
}
});
snake = new LinkedList<Point>();
snake.add(new Point(COLS/2, ROWS/2));
food = new Point();
rand = new Random();
generateFood();
isRunning = true;
while (isRunning) {
if (!isPaused) {
move();
checkCollision();
repaint();
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void move() {
Point head = snake.getFirst();
switch (direction) {
case 0:
snake.addFirst(new Point(head.x, head.y-1));
break;
case 1:
snake.addFirst(new Point(head.x+1, head.y));
break;
case 2:
snake.addFirst(new Point(head.x, head.y+1));
break;
case 3:
snake.addFirst(new Point(head.x-1, head.y));
break;
}
if (!head.equals(food)) {
snake.removeLast();
} else {
score++;
generateFood();
}
}
private void checkCollision() {
Point head = snake.getFirst();
if (head.x < 0 || head.x >= COLS || head.y < 0 || head.y >= ROWS) {
isRunning = false;
return;
}
for (int i=1; i<snake.size(); i++) {
if (head.equals(snake.get(i))) {
isRunning = false;
return;
}
}
}
private void generateFood() {
int x = rand.nextInt(COLS);
int y = rand.nextInt(ROWS);
Point p = new Point(x, y);
while (snake.contains(p)) {
x = rand.nextInt(COLS);
y = rand.nextInt(ROWS);
p = new Point(x, y);
}
food = p;
}
protected void paintComponent(Graphics g) {
super.paintComponents(g);
g.clearRect(0, 0, getWidth(), getHeight());
g.drawRect(0, 0, COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
g.drawString("Score: " + score, 10, 20);
for (Point p : snake) {
g.fillRect(p.x * BLOCK_SIZE, p.y * BLOCK_SIZE,
BLOCK_SIZE, BLOCK_SIZE);
}
g.setColor(java.awt.Color.RED);
g.fillRect(food.x * BLOCK_SIZE, food.y * BLOCK_SIZE,
BLOCK_SIZE, BLOCK_SIZE);
g.setColor(java.awt.Color.BLACK);
}
}
```
这个游戏使用 Java Swing 库来实现界面和交互。运行后,按空格键可以暂停/继续游戏,上下左右键控制蛇的移动方向。
阅读全文
相关推荐
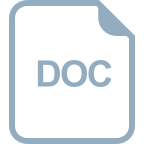
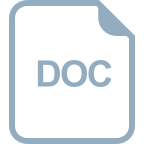
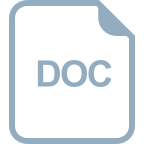









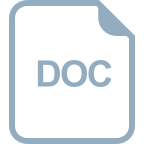
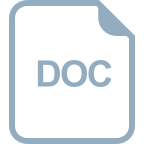
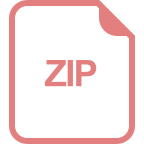
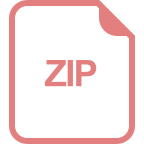
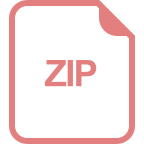
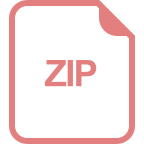
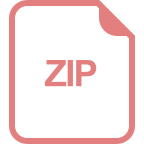
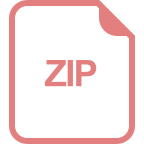