用java3.编写一段程序,实现从控制台中读取一个数字,然后打印出这个数字四舍五入后对应的人民币大写汉字字符。例如: 输入:3000 输出:叁仟 输入:300.569 输出:三佰元五角七分
时间: 2024-06-11 08:07:28 浏览: 15
```
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.Scanner;
public class Main {
private static final String[] CN_NUMBERS = {"零", "壹", "贰", "叁", "肆", "伍", "陆", "柒", "捌", "玖"};
private static final String[] CN_UNITS = {"元", "拾", "佰", "仟", "万", "拾万", "佰万", "仟万", "亿", "拾亿", "佰亿", "仟亿"};
private static final String CN_DOLLAR = "元";
private static final String CN_NEGATIVE = "负";
private static final String CN_INTEGER = "整";
private static final String CN_ZERO = "零";
private static final String CN_TEN_CENTS = "角";
private static final String CN_CENTS = "分";
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个数字:");
BigDecimal number = scanner.nextBigDecimal();
String result = convertNumberToCN(number);
System.out.println("对应的人民币大写汉字字符为:" + result);
}
private static String convertNumberToCN(BigDecimal number) {
StringBuilder sb = new StringBuilder();
if (number.compareTo(BigDecimal.ZERO) < 0) {
sb.append(CN_NEGATIVE);
number = number.abs();
}
number = number.setScale(2, RoundingMode.HALF_UP);
String[] parts = number.toString().split("\\.");
String integerPart = parts[0];
String decimalPart = parts.length > 1 ? parts[1] : "";
if (!integerPart.equals("0")) {
int unitIndex = 0;
boolean isZero = false;
for (int i = integerPart.length() - 1; i >= 0; i--) {
int digit = integerPart.charAt(i) - '0';
if (digit == 0) {
isZero = true;
if (unitIndex == 4 || unitIndex == 8) {
sb.insert(0, CN_UNITS[unitIndex]);
}
} else {
if (isZero) {
sb.insert(0, CN_ZERO);
}
sb.insert(0, CN_NUMBERS[digit]).insert(1, CN_UNITS[unitIndex]);
isZero = false;
}
unitIndex++;
}
sb.append(CN_DOLLAR);
}
if (!decimalPart.equals("")) {
int tenCents = decimalPart.charAt(0) - '0';
int cents = decimalPart.length() > 1 ? decimalPart.charAt(1) - '0' : 0;
if (tenCents + cents == 0) {
sb.append(CN_INTEGER);
} else {
if (tenCents != 0) {
sb.append(CN_NUMBERS[tenCents]).append(CN_TEN_CENTS);
}
if (cents != 0) {
sb.append(CN_NUMBERS[cents]).append(CN_CENTS);
}
}
} else {
sb.append(CN_INTEGER);
}
return sb.toString();
}
}
```
相关推荐
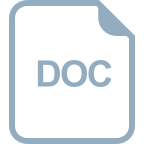
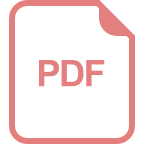
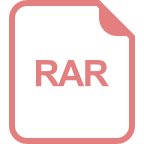





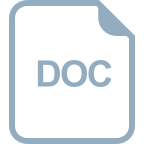
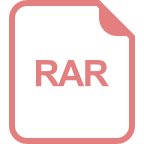
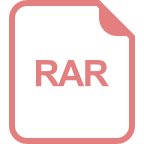
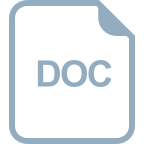
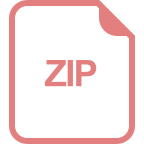
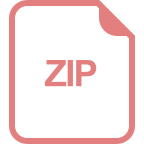
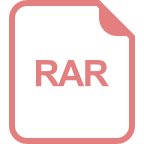
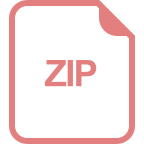
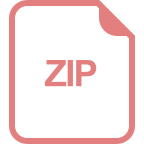