java 实现k-means
时间: 2024-05-04 22:17:26 浏览: 66
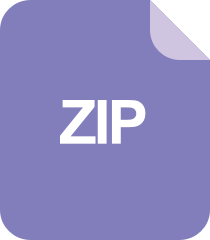
java实现k-means算法

K-means是一种聚类算法,可以通过Java实现。以下是简单的Java代码示例:
首先,定义一个Cluster类表示聚类中心:
```
public class Cluster {
private List<Point> points;
private Point centroid;
public Cluster(Point centroid) {
this.centroid = centroid;
this.points = new ArrayList<>();
}
public List<Point> getPoints() {
return points;
}
public void addPoint(Point point) {
points.add(point);
}
public void clear() {
points.clear();
}
public Point getCentroid() {
return centroid;
}
public void setCentroid(Point centroid) {
this.centroid = centroid;
}
}
```
定义一个Point类表示数据点:
```
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double distance(Point other) {
double dx = x - other.getX();
double dy = y - other.getY();
return Math.sqrt(dx * dx + dy * dy);
}
}
```
然后实现KMeans类:
```
public class KMeans {
private int k;
private List<Point> points;
private List<Cluster> clusters;
private Random random;
public KMeans(int k, List<Point> points) {
this.k = k;
this.points = points;
this.clusters = new ArrayList<>();
this.random = new Random();
}
public void run() {
// 初始化聚类中心
for (int i = 0; i < k; i++) {
Point centroid = points.get(random.nextInt(points.size()));
Cluster cluster = new Cluster(centroid);
clusters.add(cluster);
}
boolean converged = false;
while (!converged) {
// 分配数据点到最近的聚类中心
for (Point point : points) {
Cluster closest = null;
double minDistance = Double.MAX_VALUE;
for (Cluster cluster : clusters) {
double distance = point.distance(cluster.getCentroid());
if (distance < minDistance) {
closest = cluster;
minDistance = distance;
}
}
closest.addPoint(point);
}
// 更新聚类中心
converged = true;
for (Cluster cluster : clusters) {
Point oldCentroid = cluster.getCentroid();
Point newCentroid = calculateCentroid(cluster.getPoints());
if (!oldCentroid.equals(newCentroid)) {
cluster.setCentroid(newCentroid);
cluster.clear();
converged = false;
}
}
}
}
private Point calculateCentroid(List<Point> points) {
double sumX = 0;
double sumY = 0;
for (Point point : points) {
sumX += point.getX();
sumY += point.getY();
}
double centerX = sumX / points.size();
double centerY = sumY / points.size();
return new Point(centerX, centerY);
}
public List<Cluster> getClusters() {
return clusters;
}
}
```
使用方法如下:
```
List<Point> points = Arrays.asList(
new Point(1, 1),
new Point(1, 2),
new Point(2, 2),
new Point(8, 8),
new Point(9, 8),
new Point(8, 9)
);
KMeans kMeans = new KMeans(2, points);
kMeans.run();
List<Cluster> clusters = kMeans.getClusters();
for (Cluster cluster : clusters) {
System.out.println(cluster.getCentroid());
for (Point point : cluster.getPoints()) {
System.out.println(" " + point);
}
}
```
输出结果如下:
```
(1.3333333333333333, 1.6666666666666667)
(1.0, 1.0)
(1.0, 2.0)
(2.0, 2.0)
(8.666666666666666, 8.333333333333334)
(8.0, 8.0)
(9.0, 8.0)
(8.0, 9.0)
```
其中,第一组数据点被分到了一个聚类中心,第二组数据点被分到了另一个聚类中心。这就是K-means聚类的结果。
阅读全文
相关推荐
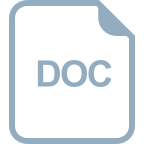
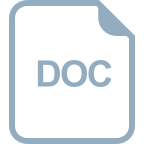
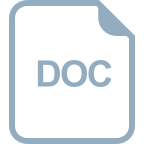
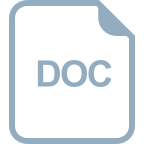



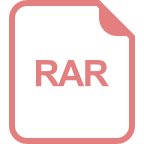
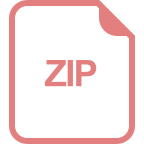
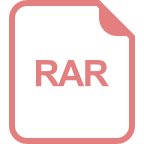
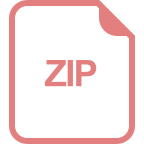
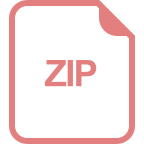

