dlib 计算面部朝向 示例
时间: 2024-02-04 22:03:36 浏览: 19
好的,我能回答您的问题。以下是一个使用dlib计算面部朝向的示例代码:
```python
import dlib
import cv2
import numpy as np
# 加载模型
predictor_path = "shape_predictor_68_face_landmarks.dat"
predictor = dlib.shape_predictor(predictor_path)
# 初始化人脸检测器
detector = dlib.get_frontal_face_detector()
# 读取图像
img = cv2.imread("test.jpg")
# 灰度转换
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 人脸检测
faces = detector(gray, 1)
for face in faces:
# 特征点检测
landmarks = predictor(gray, face)
landmarks = np.array([(landmarks.part(i).x, landmarks.part(i).y) for i in range(68)])
# 计算面部朝向
image_points = np.array([
landmarks[30], # 鼻尖
landmarks[8], # 下巴
landmarks[36], # 左眼角
landmarks[45], # 右眼角
landmarks[48], # 嘴左角
landmarks[54] # 嘴右角
], dtype="double")
model_points = np.array([
(0.0, 0.0, 0.0),
(0.0, -330.0, -65.0),
(-225.0, 170.0, -135.0),
(225.0, 170.0, -135.0),
(-150.0, -150.0, -125.0),
(150.0, -150.0, -125.0)
])
focal_length = img.shape[1]
center = (img.shape[1]/2, img.shape[0]/2)
camera_matrix = np.array([
[focal_length, 0, center[0]],
[0, focal_length, center[1]],
[0, 0, 1]
], dtype="double")
dist_coeffs = np.zeros((4,1))
success, rotation_vector, translation_vector = cv2.solvePnP(model_points, image_points, camera_matrix, dist_coeffs, flags=cv2.SOLVEPNP_ITERATIVE)
nose_end_point2D, jacobian = cv2.projectPoints(np.array([(0.0, 0.0, 1000.0)]), rotation_vector, translation_vector, camera_matrix, dist_coeffs)
p1 = (int(image_points[0][0]), int(image_points[0][1]))
p2 = (int(nose_end_point2D[0][0][0]), int(nose_end_point2D[0][0][1]))
cv2.line(img, p1, p2, (0, 255, 0), 2)
# 显示结果图像
cv2.imshow("Output", img)
cv2.waitKey(0)
```
此代码使用dlib库来检测面部朝向,首先需要加载预训练好的特征点检测器。然后使用人脸检测器检测图像中的人脸,然后计算每个人脸的面部朝向。最后,将结果绘制在原始图像上并显示。
相关推荐
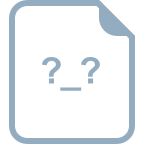
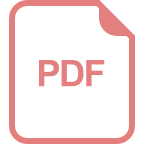














