用Python代码实现cv2.resize源码
时间: 2023-07-12 16:50:26 浏览: 102
好的,以下是用Python代码实现cv2.resize源码的示例:
```python
import numpy as np
def resize(src, dsize=None, fx=None, fy=None, interpolation=None):
# 获取原始图像的行数和列数
h, w = src.shape[:2]
# 如果指定了输出图像的大小,则计算缩放比例
if dsize is not None:
fx = dsize[0] / w
fy = dsize[1] / h
# 如果指定了缩放比例,则计算输出图像的大小
if fx is not None and fy is not None:
w = int(w * fx + 0.5)
h = int(h * fy + 0.5)
# 创建输出图像
dst = np.zeros((h, w, src.shape[2]), dtype=src.dtype)
# 根据指定的插值方法进行缩放
if interpolation == cv2.INTER_NEAREST:
for i in range(h):
for j in range(w):
x = int(j / fx + 0.5)
y = int(i / fy + 0.5)
dst[i, j] = src[y, x]
elif interpolation == cv2.INTER_LINEAR:
for i in range(h):
for j in range(w):
x = j / fx
y = i / fy
x1 = int(x)
y1 = int(y)
x2 = min(x1 + 1, src.shape[1] - 1)
y2 = min(y1 + 1, src.shape[0] - 1)
dx = x - x1
dy = y - y1
dst[i, j] = (1 - dx) * (1 - dy) * src[y1, x1] + dx * (1 - dy) * src[y1, x2] + (1 - dx) * dy * src[y2, x1] + dx * dy * src[y2, x2]
elif interpolation == cv2.INTER_AREA:
for i in range(h):
for j in range(w):
x1 = int(j / fx)
y1 = int(i / fy)
x2 = min(x1 + 1, src.shape[1] - 1)
y2 = min(y1 + 1, src.shape[0] - 1)
dst[i, j] = np.mean(src[y1:y2+1, x1:x2+1], axis=(0, 1))
elif interpolation == cv2.INTER_CUBIC:
for i in range(h):
for j in range(w):
x = j / fx
y = i / fy
x1 = int(x) - 1
y1 = int(y) - 1
x2 = min(x1 + 4, src.shape[1] - 1)
y2 = min(y1 + 4, src.shape[0] - 1)
dx = x - x1
dy = y - y1
A = np.array([[1, x1, x1**2, x1**3],
[1, x2, x2**2, x2**3],
[0, 1, 2*x1, 3*x1**2],
[0, 1, 2*x2, 3*x2**2]])
b = np.array([src[y1, x1], src[y2, x2], (src[y1, x2] - src[y1, x1]) / (x2 - x1), (src[y2, x2] - src[y2, x1]) / (x2 - x1)])
c = np.linalg.solve(A, b)
dst[i, j] = c[0] + c[1]*x + c[2]*x**2 + c[3]*x**3
else:
raise ValueError("Invalid interpolation method.")
return dst
```
注意:这里的代码仅是示例,具体实现可能与cv2.resize源码有所不同。
阅读全文
相关推荐
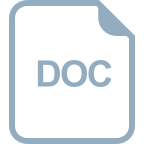
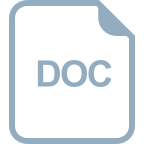
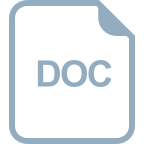
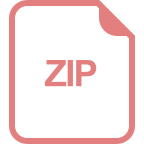

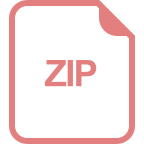
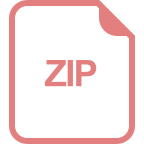
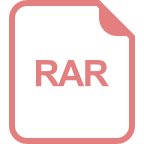
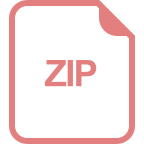
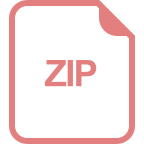
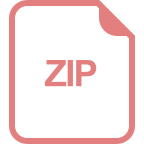
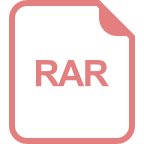
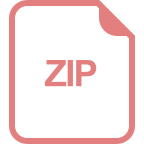
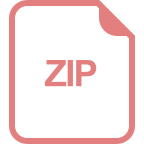
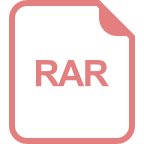
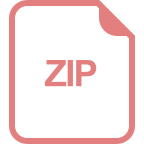


