python统计列表中词频
时间: 2023-09-26 13:09:02 浏览: 39
可以使用Python中的collections模块来统计列表中每个元素出现的次数。具体实现可以使用Counter类。
示例代码如下:
```python
from collections import Counter
my_list = ["apple", "banana", "apple", "cherry", "banana", "apple"]
word_count = Counter(my_list)
print(word_count)
```
输出结果:
```
Counter({'apple': 3, 'banana': 2, 'cherry': 1})
```
其中,Counter函数返回一个字典,键为列表中的元素,值为该元素在列表中出现的次数。
相关问题
python统计文本文件词频
在Python中,你可以使用`collections`模块中的`Counter`类来统计文本文件中的词频。这里是一个简单的步骤:
1. 首先,你需要打开并读取文本文件。可以使用内置的`open()`函数,并指定模式为'r'(读取)。
```python
with open('filename.txt', 'r', encoding='utf-8') as file:
text = file.read()
```
2. 接着,将文本分割成单词列表。通常我们会去除标点符号、转换为小写等预处理操作,然后使用`split()`方法。
```python
import string
words = text.translate(str.maketrans('', '', string.punctuation)).lower().split()
```
3. 使用`Counter`计算每个单词的频率。
```python
word_count = Counter(words)
```
4. 最后,你可以遍历这个字典,打印出最常见的单词及其频率。
```python
for word, freq in word_count.most_common():
print(f'{word}: {freq}')
```
如果你想保存结果到文件中,可以用`json`或`pickle`模块将其序列化。
python中文词频统计_Python中文词频统计
在Python中进行中文词频统计,可以使用jieba库来进行中文分词。以下是一个简单的示例:
```python
import jieba
# 读取文本文件
with open("text.txt", "r", encoding="utf-8") as f:
text = f.read()
# 使用jieba分词
words = jieba.cut(text)
# 统计词频
freq = {}
for word in words:
if word in freq:
freq[word] += 1
else:
freq[word] = 1
# 输出结果
for word, count in freq.items():
print(word, count)
```
其中,先使用`jieba.cut()`方法对文本进行分词,然后使用一个字典来统计每个词出现的次数。最后遍历字典,输出结果。需要注意的是,在使用jieba库前,需要先安装和下载其所需要的字典文件。
阅读全文
相关推荐
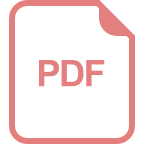
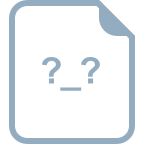







