python统计csv词频
时间: 2023-06-29 15:20:46 浏览: 72
可以使用Python的csv模块和collections模块来统计csv文件中的词频。
下面是一个简单的示例代码:
```python
import csv
from collections import Counter
with open('file.csv', 'r') as file:
reader = csv.reader(file)
words = [word for line in reader for word in line] # 将csv文件中的所有单词存储到一个列表中
word_counts = Counter(words) # 统计单词出现次数
print(word_counts.most_common(10)) # 打印出现次数最多的前10个单词及其出现次数
```
其中,`file.csv`是你要统计词频的csv文件名。你可以将上述代码中的`10`修改成你想要显示的前几个单词即可。
相关问题
统计csv词频_Jieba统计词频并生成csv文件
好的,我可以为您提供Jieba统计词频并生成csv文件的代码。请确认您已经安装了Jieba库和pandas库。
```python
import jieba
import pandas as pd
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用Jieba进行分词
words = jieba.cut(text)
# 统计词频并生成DataFrame
word_freq = {}
for word in words:
if len(word) > 1: # 只统计长度大于1的词语
word_freq[word] = word_freq.get(word, 0) + 1
df = pd.DataFrame(list(word_freq.items()), columns=['词语', '词频'])
# 保存为csv文件
df.to_csv('word_freq.csv', index=False, encoding='utf-8-sig')
```
在上面的代码中,假设您要统计的文本文件名为`text.txt`,代码将会对该文本进行分词并统计每个词语的词频,最终将结果保存为一个名为`word_freq.csv`的csv文件。您可以根据实际情况修改文件名和路径。
python对csv进行词频统计
好的,针对您的问题,您可以使用Python的csv和collections库来进行词频统计。具体代码如下:
```python
import csv
from collections import Counter
with open('example.csv','r', encoding='utf-8') as f: #将"example.csv"替换成你要读取的csv文件名
reader = csv.reader(f)
content = [row for row in reader]
words = []
for row in content:
words += row
word_counts = Counter(words)
print(word_counts.most_common())
```
以上代码可以读取csv文件中的所有内容,并使用Counter函数统计词频并输出结果。希望这个代码对您有所帮助。
阅读全文
相关推荐
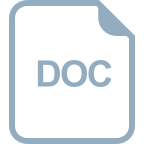
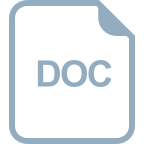
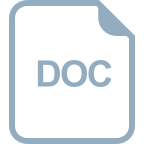








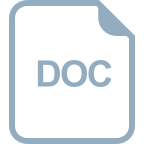
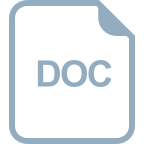
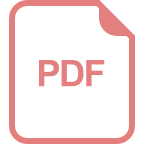
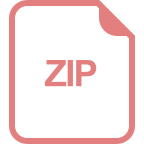
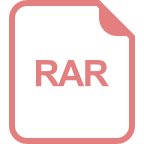
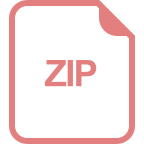