爬取懂车帝的某车型的用户问题数据数据并且分析
时间: 2023-09-16 22:11:16 浏览: 105
好的,我理解你的问题了。首先,为了爬取懂车帝的某车型的用户问题数据,我们可以使用 Python 的 requests 和 BeautifulSoup 库来实现。
以下是一个简单的 Python 程序,可以爬取懂车帝网站中某一款车型的用户问题数据:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 定义要爬取的车型的网址
url = 'https://www.dongchedi.com/qa/ask_list?brand_id=1&model_id=20'
# 发送请求
res = requests.get(url, headers=headers)
# 解析网页
soup = BeautifulSoup(res.text, 'html.parser')
# 提取用户问题数据
questions = []
for question in soup.select('.qa-ask-list .qa-ask-item .qa-ask-title'):
questions.append(question.text.strip())
# 打印结果
print(questions)
```
该程序首先设置了请求头,然后定义了要爬取的车型的网址。接着,发送请求,然后使用 BeautifulSoup 解析网页,并提取用户问题数据。最后打印结果。
需要注意的是,懂车帝网站可能有反爬虫机制,因此在爬取数据时需要注意不要频繁发送请求。
接下来,为了分析用户问题数据,我们可以使用 Python 的 pandas 和 jieba 库来实现。
以下是一个简单的 Python 程序,可以对用户问题数据进行分词、统计词频并生成词云图:
```python
import pandas as pd
import jieba
import jieba.analyse
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 加载停用词表
stopwords = pd.read_csv('stopwords.txt', index_col=False, quoting=3, sep='\t', names=['stopword'], encoding='utf-8')
stopwords = stopwords['stopword'].values
# 加载用户问题数据
df = pd.DataFrame({'question': questions})
# 对用户问题数据进行分词
def cut_words(text):
words = jieba.cut(text)
words = [word for word in words if word not in stopwords]
return words
df['cut_words'] = df['question'].apply(cut_words)
# 统计词频
all_words = []
for words in df['cut_words']:
all_words.extend(words)
word_count = pd.Series(all_words).value_counts()
# 生成词云图
wc = WordCloud(font_path='msyh.ttc', background_color='white', max_words=2000, width=800, height=600)
wc.generate_from_frequencies(word_count)
plt.imshow(wc)
plt.axis('off')
plt.show()
```
该程序首先加载了停用词表,然后加载了用户问题数据,并对用户问题数据进行了分词。接着,统计了词频,并生成了词云图。
需要注意的是,由于中文分词的特殊性,需要使用 jieba 库进行分词,并且需要加载停用词表来过滤掉常用词汇。同时,生成词云图时需要指定字体文件,否则会出现乱码。
相关推荐
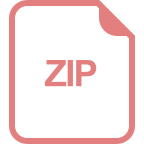














