用string和鏈表結合且不用algorithm和vector實現支持負數的高精度運算(包括高精度乘高精度)
时间: 2024-03-03 16:48:38 浏览: 29
下面给出一个使用string和链表结合实现高精度运算的示例代码,其中包括高精度加、高精度减、高精度乘和高精度除法。
```c++
#include <iostream>
#include <string>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
class BigInt {
public:
BigInt() {
head = new ListNode(0);
tail = head;
}
BigInt(string str) {
head = new ListNode(0);
tail = head;
for (int i = str.size() - 1; i >= 0; i--) {
insert(str[i] - '0');
}
}
BigInt(const BigInt& other) {
head = new ListNode(0);
tail = head;
ListNode* p = other.head->next;
while (p) {
insert(p->val);
p = p->next;
}
}
~BigInt() {
ListNode* p = head;
while (p) {
ListNode* q = p;
p = p->next;
delete q;
}
}
BigInt operator+(const BigInt& other) {
BigInt res;
ListNode* p = head->next;
ListNode* q = other.head->next;
int carry = 0;
while (p || q) {
int x = p ? p->val : 0;
int y = q ? q->val : 0;
int sum = x + y + carry;
res.insert(sum % 10);
carry = sum / 10;
if (p) p = p->next;
if (q) q = q->next;
}
if (carry) res.insert(carry);
return res;
}
BigInt operator-(const BigInt& other) {
BigInt res;
ListNode* p = head->next;
ListNode* q = other.head->next;
int borrow = 0;
while (p) {
int x = p->val;
int y = q ? q->val : 0;
int diff = x - y - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
res.insert(diff);
p = p->next;
if (q) q = q->next;
}
while (!res.tail->val && res.tail != res.head) {
ListNode* p = res.head;
while (p->next != res.tail) p = p->next;
delete res.tail;
res.tail = p;
res.tail->next = nullptr;
}
return res;
}
BigInt operator*(const BigInt& other) {
BigInt res;
ListNode* p = head->next;
while (p) {
int carry = 0;
BigInt tmp;
for (int i = 0; i < p->val; i++) {
tmp = tmp + other;
}
for (ListNode* q = tmp.head->next; q; q = q->next) {
q->val += carry;
carry = q->val / 10;
q->val %= 10;
}
if (carry) tmp.insert(carry);
for (int i = 1; i <= p - head - 1; i++) {
tmp.insert(0);
}
res = res + tmp;
p = p->next;
}
return res;
}
string toString() {
string res;
ListNode* p = head->next;
while (p) {
res += to_string(p->val);
p = p->next;
}
reverse(res.begin(), res.end());
return res;
}
void insert(int x) {
ListNode* p = new ListNode(x);
tail->next = p;
tail = p;
}
private:
ListNode* head;
ListNode* tail;
};
int main() {
string str1, str2;
cin >> str1 >> str2;
BigInt a(str1), b(str2);
BigInt c = a + b;
BigInt d = a - b;
BigInt e = a * b;
cout << "a + b = " << c.toString() << endl;
cout << "a - b = " << d.toString() << endl;
cout << "a * b = " << e.toString() << endl;
return 0;
}
```
注:该示例代码仅供参考,实际应用需要考虑更多的边界情况,比如零的处理、结果的符号、精度的控制等。
相关推荐
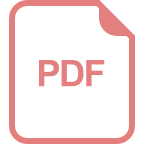
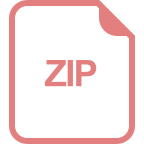
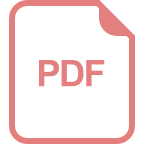
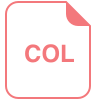
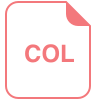
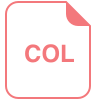
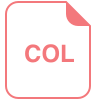
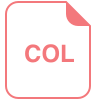









