通过java代码实现数组[2,5,6,8] 降序排列
时间: 2024-05-14 19:12:16 浏览: 7
可以使用Arrays类中的sort方法,传入降序排列的Comparator来实现:
```java
import java.util.Arrays;
import java.util.Comparator;
public class Main {
public static void main(String[] args) {
int[] arr = {2, 5, 6, 8};
Integer[] newArr = new Integer[arr.length];
for (int i = 0; i < arr.length; i++) {
newArr[i] = arr[i];
}
Arrays.sort(newArr, Comparator.reverseOrder());
System.out.println(Arrays.toString(newArr));
}
}
```
输出:
```
[8, 6, 5, 2]
```
首先将原数组转换为Integer类型的数组,然后使用Arrays类中的sort方法,传入Comparator.reverseOrder()来实现降序排列。最后输出排好序的数组。
相关问题
用java把int数组按照降序排列代码,用到sort和comparator
以下是使用 Java 中的 sort 和 Comparator 接口将 int 数组按照降序排列的代码示例:
```java
import java.util.Arrays;
import java.util.Comparator;
public class Main {
public static void main(String[] args) {
int[] arr = { 3, 5, 1, 4, 2 };
// 使用 Arrays.sort 函数,降序排列数组
Arrays.sort(arr, new Comparator<Integer>() {
@Override
public int compare(Integer a, Integer b) {
return b - a;
}
});
// 输出排序后的数组
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
```
输出结果为:5 4 3 2 1
用java把double数组按照降序排列代码,用到sort和comparator
以下是使用 Java 中的 sort 和 Comparator 接口将 double 数组按照降序排列的代码示例:
```java
import java.util.Arrays;
import java.util.Comparator;
public class Main {
public static void main(String[] args) {
double[] arr = { 3.2, 5.7, 1.4, 4.1, 2.9 };
// 使用 Arrays.sort 函数,降序排列数组
Arrays.sort(arr, new Comparator<Double>() {
@Override
public int compare(Double a, Double b) {
return Double.compare(b, a);
}
});
// 输出排序后的数组
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
```
输出结果为:5.7 4.1 3.2 2.9 1.4
相关推荐
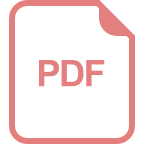
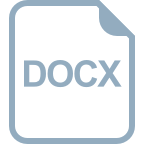












