如果自定义了一个点的类,我如何能用java让其中的元素进行加减乘除运算
时间: 2024-10-08 18:14:43 浏览: 25
在Java中,你可以为自定义的点类定义一些操作方法,如加法、减法、乘法和除法,以便对点的坐标进行数学运算。以下是一个简单的示例:
```java
public class Point {
private double x;
private double y;
// 构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 获取坐标
public double getX() { return x; }
public double getY() { return y; }
// 设置坐标
public void setX(double x) { this.x = x; }
public void setY(double y) { this.y = y; }
// 加法运算
public Point add(Point other) {
return new Point(this.x + other.getX(), this.y + other.getY());
}
// 减法运算
public Point subtract(Point other) {
return new Point(this.x - other.getX(), this.y - other.getY());
}
// 乘法运算(通常表示缩放)
public Point multiply(double scaleFactor) {
return new Point(this.x * scaleFactor, this.y * scaleFactor);
}
// 除法运算(除以非零数,若其他点为原点则特殊处理)
public Point divide(double divisor) throws ArithmeticException {
if (divisor != 0) {
return new Point(this.x / divisor, this.y / divisor);
} else {
throw new ArithmeticException("Cannot divide by zero");
}
}
}
```
现在,你可以实例化两个点对象,然后通过它们的方法进行运算:
```java
Point p1 = new Point(1, 2);
Point p2 = new Point(3, 4);
Point sum = p1.add(p2); // 结果是新的点(4, 6)
Point difference = p1.subtract(p2); // 结果是新的点(-2, -2)
Point scaled = p1.multiply(2); // 缩小两倍的新点(2, 4)
try {
Point quotient = p1.divide(2); // 结果是新点(0.5, 1)
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
```
阅读全文
相关推荐


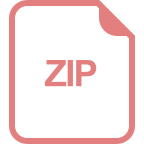
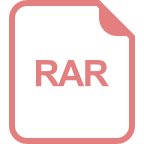
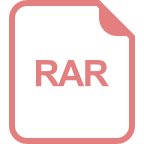













