双线性插值c语言代码
时间: 2024-09-09 22:02:47 浏览: 29
双线性插值是一种常见的数值计算方法,在计算机图形学、图像处理等领域广泛应用,用于估算给定二维网格中某个点的值。在C语言中,你可以通过以下步骤编写简单的双线性插值函数:
```c
#include <stdio.h>
#include <float.h>
// 定义一个二维数组
typedef struct {
float x;
float y;
float z; // 给定网格的数据值
} Point;
// 双线性插值函数
float bilinear_interpolation(Point* points, int rows, int cols, float x, float y) {
if (x < 0 || x >= cols || y < 0 || y >= rows) {
printf("Out of bounds!\n");
return DBL_MIN; // 返回边界之外的值,通常是浮点最小值
}
int row = y;
int col = x;
float u = (x - row);
// 计算四个邻居的z值
float p00 = points[row * cols + col].z;
float p01 = points[(row + 1) * cols + col].z;
float p10 = points[row * cols + (col + 1)].z;
float p11 = points[(row + 1) * cols + (col + 1)].z;
// 双线性插值公式
return p00 * (1 - v) + p10 * (1 - u) * v + p11 * u * v;
}
int main() {
// 创建一个二维数组示例
Point points[4] = { {0, 0, 1}, {1, 0, 2}, {0, 1, 3}, {1, 1, 4} };
int rows = 2;
int cols = 2;
float x = 0.5;
float y = 0.5;
float interpolated_z = bilinear_interpolation(points, rows, cols, x, y);
printf("Interpolated value at (%f, %f) is: %f\n", x, y, interpolated_z);
return 0;
}
```
在这个例子中,`bilinear_interpolation`函数接收一个二维点数组、行数和列数以及查询的坐标(x, y),返回对应位置的插值结果。
相关推荐
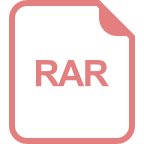
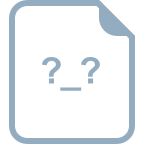














