使用C语言 图像缩放 双线性插值算法
时间: 2023-09-12 19:03:52 浏览: 255
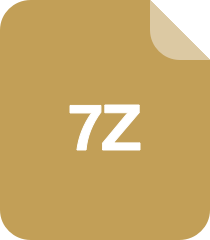
易语言源码线性插值算法实现图像缩放旋转祥解.7z
双线性插值算法是一种常用的图像缩放方法,可以在缩放过程中保持图像的平滑性和细节信息。下面是使用C语言实现双线性插值算法的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define CHANNELS 3 // 图像通道数
#define SCALE 2 // 缩放倍数
// 双线性插值算法
void bilinear_interpolation(unsigned char *input, unsigned char *output, int width, int height, int channels, float scale) {
int new_width = (int)(width * scale);
int new_height = (int)(height * scale);
float x_ratio = (float)(width - 1) / (float)(new_width - 1);
float y_ratio = (float)(height - 1) / (float)(new_height - 1);
for (int i = 0; i < new_height; i++) {
for (int j = 0; j < new_width; j++) {
float x = j * x_ratio;
float y = i * y_ratio;
int x1 = (int)x;
int y1 = (int)y;
int x2 = x1 + 1;
int y2 = y1 + 1;
float fx = x - x1;
float fy = y - y1;
for (int k = 0; k < channels; k++) {
float p1 = input[(y1 * width + x1) * channels + k];
float p2 = input[(y1 * width + x2) * channels + k];
float p3 = input[(y2 * width + x1) * channels + k];
float p4 = input[(y2 * width + x2) * channels + k];
float value = (1 - fx) * (1 - fy) * p1 + fx * (1 - fy) * p2 + (1 - fx) * fy * p3 + fx * fy * p4;
output[(i * new_width + j) * channels + k] = (unsigned char)value;
}
}
}
}
int main() {
FILE *fp_in, *fp_out;
unsigned char *input, *output;
int width, height;
fp_in = fopen("input.bmp", "rb");
if (fp_in == NULL) {
printf("Error: cannot open input file!\n");
return -1;
}
// 读取BMP文件头
fseek(fp_in, 18, SEEK_SET);
fread(&width, sizeof(int), 1, fp_in);
fread(&height, sizeof(int), 1, fp_in);
// 计算图像数据大小
int size = width * height * CHANNELS;
// 分配内存并读取图像数据
input = (unsigned char*)malloc(size);
fread(input, sizeof(unsigned char), size, fp_in);
// 缩放图像
int new_width = (int)(width * SCALE);
int new_height = (int)(height * SCALE);
output = (unsigned char*)malloc(new_width * new_height * CHANNELS);
bilinear_interpolation(input, output, width, height, CHANNELS, SCALE);
// 写入输出文件
fp_out = fopen("output.bmp", "wb");
if (fp_out == NULL) {
printf("Error: cannot open output file!\n");
return -1;
}
fwrite(input, sizeof(unsigned char), size, fp_out);
fclose(fp_out);
// 释放内存
free(input);
free(output);
return 0;
}
```
上面的代码使用双线性插值算法实现了图像缩放,输入图像为BMP格式,输出图像也是BMP格式。在实际应用中,还需要考虑图像的边缘处理、颜色空间转换等问题。
阅读全文
相关推荐


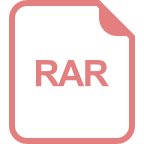
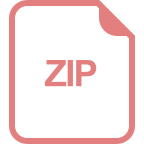
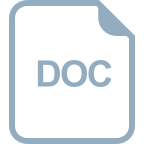
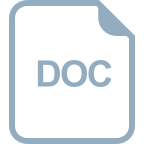


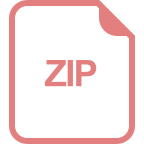
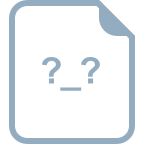
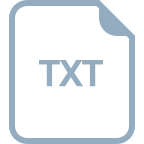
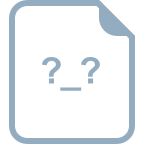
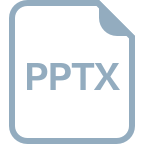
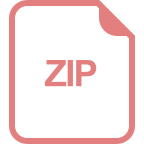