双线性插值算法 C++
时间: 2023-09-12 13:03:49 浏览: 115
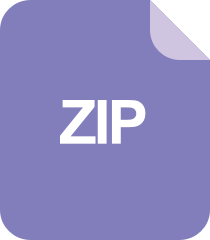
双线性插值算法c++
双线性插值算法是一种常用的图像缩放算法,可以通过对原始图像进行插值计算,生成指定大小的新图像。以下是双线性插值算法的C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 640 // 原始图像宽度
#define HEIGHT 480 // 原始图像高度
typedef struct {
int r, g, b;
} RGB;
RGB img[HEIGHT][WIDTH]; // 原始图像数据
RGB newimg[2*HEIGHT][2*WIDTH]; // 生成的新图像数据
// 双线性插值计算
RGB bilinear_interp(RGB p11, RGB p12, RGB p21, RGB p22, double x, double y) {
RGB r1, r2, p;
double fx1, fx2;
fx1 = (1 - x) * p11.r + x * p21.r;
fx2 = (1 - x) * p12.r + x * p22.r;
r1.r = (int) ((1 - y) * fx1 + y * fx2 + 0.5);
fx1 = (1 - x) * p11.g + x * p21.g;
fx2 = (1 - x) * p12.g + x * p22.g;
r1.g = (int) ((1 - y) * fx1 + y * fx2 + 0.5);
fx1 = (1 - x) * p11.b + x * p21.b;
fx2 = (1 - x) * p12.b + x * p22.b;
r1.b = (int) ((1 - y) * fx1 + y * fx2 + 0.5);
return r1;
}
// 双线性插值缩放
void bilinear_scale(int w, int h, int nw, int nh) {
double x_ratio = (double)(w - 1) / nw;
double y_ratio = (double)(h - 1) / nh;
for (int i = 0; i < nh; i++) {
for (int j = 0; j < nw; j++) {
int x = (int)(x_ratio * j);
int y = (int)(y_ratio * i);
double x_diff = (x_ratio * j) - x;
double y_diff = (y_ratio * i) - y;
RGB p11 = img[y][x];
RGB p12 = img[y][x+1];
RGB p21 = img[y+1][x];
RGB p22 = img[y+1][x+1];
newimg[i*2][j*2] = p11;
newimg[i*2][j*2+1] = bilinear_interp(p11, p12, p21, p22, x_diff, 0);
newimg[i*2+1][j*2] = bilinear_interp(p11, p12, p21, p22, 0, y_diff);
newimg[i*2+1][j*2+1] = bilinear_interp(p11, p12, p21, p22, x_diff, y_diff);
}
}
}
int main() {
// 读取原始图像数据
FILE *fp = fopen("lena.rgb", "rb");
fread(img, sizeof(RGB), WIDTH*HEIGHT, fp);
fclose(fp);
// 双线性插值缩放
bilinear_scale(WIDTH, HEIGHT, WIDTH*2, HEIGHT*2);
// 保存生成的新图像
fp = fopen("lena2x.rgb", "wb");
fwrite(newimg, sizeof(RGB), WIDTH*2*HEIGHT*2, fp);
fclose(fp);
return 0;
}
```
其中,`RGB`结构体表示一个像素点的RGB颜色值,`img`数组存储原始图像数据,`newimg`数组存储生成的新图像数据。`bilinear_interp`函数实现双线性插值计算,`bilinear_scale`函数实现双线性插值缩放。
在主函数中,首先读取原始图像数据,然后调用`bilinear_scale`函数进行双线性插值缩放,最后保存生成的新图像数据。
阅读全文
相关推荐
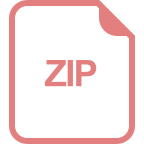















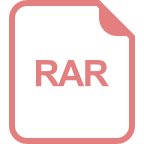