c++函数重载运算符
时间: 2023-08-13 14:39:13 浏览: 664
C++中的函数重载运算符允许我们对内置运算符(如加号、减号等)进行自定义操作。函数重载运算符的格式为:
```
返回类型 operator 运算符 (参数列表) {
// 函数体
}
```
其中,`operator`关键字后面跟着要重载的运算符,参数列表中的参数个数和类型应该与该运算符的操作数相对应,返回类型可以是任何类型,包括自定义类型。
举个例子,我们可以定义一个`Complex`类,重载加号运算符,实现复数的加法操作:
```c++
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator + (const Complex& c) {
return Complex(real + c.real, imag + c.imag);
}
private:
double real;
double imag;
};
```
这样,我们就可以像使用内置类型一样使用`Complex`类型了:
```c++
Complex a(1.0, 2.0);
Complex b(3.0, 4.0);
Complex c = a + b; // 调用重载的加号运算符,c的值为(4.0, 6.0)
```
相关问题
c++重载判断运算符
在 C++ 中,重载运算符允许程序员自定义基本数学、逻辑或其他预定义的操作,使其能够应用于特定的数据类型。当涉及到比较、算术、逻辑等运算时,你可以创建函数模板或者成员函数来“重载”标准库提供的运算符。
例如,如果你有一个名为 `Point` 的类,你可以重载 `+` 运算符使得两个点可以相加,或者 `==` 运算符来比较两个点是否相等。重载运算符需要遵守一些规则:
1. 函数名必须与运算符匹配,比如 `operator+(const Point &a, const Point &b)` 用于定义加法操作。
2. 返回类型通常也是该运算符对应的结果类型。
3. 参数列表应该反映出运算符的结合性和优先级。
例如:
```cpp
class Point {
public:
// 重载加法运算符
Point operator+(const Point &other) {
return {x + other.x, y + other.y};
}
// 重载等于运算符
bool operator==(const Point &other) const {
return x == other.x && y == other.y;
}
private:
int x, y;
};
// 使用示例
Point p1(0, 0), p2(1, 1);
if (p1 + p2 == Point(1, 1)) { // 等于运算符重载后的比较
std::cout << "Points are equal.\n";
}
```
c++使用重载运算符输出类
好的,我了解了您的问题。为了使用重载运算符输出类,您需要在该类中定义一个名为 operator<< 的函数,并在该函数中以所需的格式输出类的各个成员变量。例如:
```
class MyClass {
public:
int x;
std::string y;
friend std::ostream& operator<<(std::ostream& os, const MyClass& obj) {
os << "x: " << obj.x << ", y: " << obj.y;
return os;
}
};
```
然后,您就可以使用 std::cout 或其他输出流来输出 MyClass 的对象,例如:
```
MyClass obj{42, "hello"};
std::cout << obj << std::endl; // 输出:x: 42, y: hello
```
希望这可以帮助您!
阅读全文
相关推荐
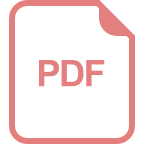
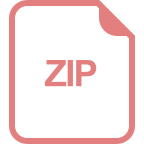
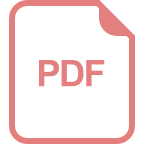













