pythonopencv,将1.jpg和2.jpg拼接/融合成一张图片,并保存为output.jpg 1. 偏移量通过特征点匹配自动计算 2. 拼接后空白区域用黑色代替
时间: 2024-03-13 11:43:28 浏览: 89
好的,我可以为您提供Python OpenCV代码来实现这个任务。您可以按照以下步骤进行操作:
1. 导入必要的库
```python
import cv2
import numpy as np
```
2. 读取两张图片
```python
img1 = cv2.imread('1.jpg')
img2 = cv2.imread('2.jpg')
```
3. 计算关键点和描述符
```python
# 初始化ORB检测器
orb = cv2.ORB_create()
# 检测关键点和描述符
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# 初始化BFMatcher
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 匹配描述符
matches = bf.match(des1, des2)
# 排序匹配项
matches = sorted(matches, key=lambda x: x.distance)
# 保留前100个匹配项
matches = matches[:100]
# 提取匹配项的关键点
src_pts = np.float32([kp1[m.queryIdx].pt for m in matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in matches]).reshape(-1, 1, 2)
# 计算透视变换矩阵
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
```
4. 计算偏移量并拼接图像
```python
# 计算图像1的宽度和高度
h1, w1 = img1.shape[:2]
# 计算图像2的宽度和高度
h2, w2 = img2.shape[:2]
# 计算图像2相对于图像1的偏移量
offset_x, offset_y = np.dot(M, np.array([w2, h2, 1]))
# 将偏移量转换为整数
offset_x, offset_y = int(offset_x), int(offset_y)
# 创建拼接后的画布
result_height = max(h1, h2 + offset_y)
result_width = max(w1, w2 + offset_x)
result = np.zeros((result_height, result_width, 3), dtype=np.uint8)
# 将图像1复制到结果画布中
result[:h1, :w1] = img1
# 将图像2复制到结果画布中
result[offset_y:h2+offset_y, offset_x:w2+offset_x] = img2
```
5. 保存结果图像
```python
cv2.imwrite('output.jpg', result)
```
这样就可以将两张图片拼接/融合成一张图片,并保存为output.jpg。其中,偏移量是通过特征点匹配自动计算的,拼接后空白区域用黑色代替。希望能对您有所帮助!
阅读全文
相关推荐


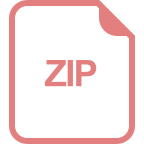

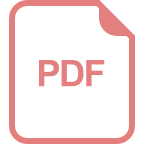
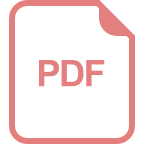
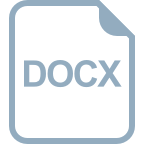
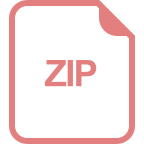