indices = [(i, i + (time_step + predict_time)) for i in range(X.shape[0] - ( time_step + predict_time) + 1)]
时间: 2024-04-29 21:19:27 浏览: 6
这段代码的作用是生成一个由元组组成的列表,列表中的每个元组都包含了两个整数,分别表示数据集中一个样本的起始索引和结束索引。其中,起始索引为 i,结束索引为 i + (time_step + predict_time)。具体来说,这段代码使用了列表生成式,通过遍历一个 range 对象来生成元组并添加到列表中,其中的 range 对象的长度为 X.shape[0] - (time_step + predict_time) + 1,即数据集中可以提取出的样本数目。
相关问题
写一个python程序使用DQN的方法解决CartPole-V0问题,使用英文注释,不使用keras
Sure, I can help you with that! Here is a simple Python program using the DQN method to solve the CartPole-V0 problem. This program does not use Keras and has English comments to help you understand what's happening.
First, we'll import the necessary libraries:
```python
import random
import numpy as np
import tensorflow as tf
import gym
```
Next, we'll set up our parameters:
```python
# Define some hyperparameters
batch_size = 32 # How many memory samples to train on
replay_memory_size = 10000 # How many recent frames to remember
gamma = 0.99 # Discount rate for future rewards
n_episodes = 5000 # How many episodes to train for
n_steps = 200 # Maximum number of steps in each episode
start_epsilon = 1.0 # Initial value of epsilon for epsilon-greedy exploration
end_epsilon = 0.05 # Final value of epsilon for epsilon-greedy exploration
epsilon_decay_steps = 10000 # Number of steps to decay epsilon from start to end value
learning_rate = 0.001 # Learning rate for the neural network optimizer
```
Now we can define our neural network:
```python
# Define the neural network
n_inputs = 4 # Number of input features (four observations from the environment)
n_hidden = 32 # Number of hidden neurons in the network
n_outputs = 2 # Number of output neurons (actions: move left or right)
initializer = tf.random_normal_initializer()
# Define the input and output placeholders
tf.reset_default_graph()
X = tf.placeholder(tf.float32, shape=[None, n_inputs])
y = tf.placeholder(tf.float32, shape=[None, n_outputs])
# Define the network architecture
hidden = tf.layers.dense(X, n_hidden, activation=tf.nn.relu, kernel_initializer=initializer)
logits = tf.layers.dense(hidden, n_outputs, kernel_initializer=initializer)
# Define the loss function and optimizer
cross_entropy = tf.nn.softmax_cross_entropy_with_logits_v2(labels=y, logits=logits)
optimizer = tf.train.AdamOptimizer(learning_rate)
training_op = optimizer.minimize(cross_entropy)
# Define the prediction and exploration functions
predict_op = tf.argmax(logits, axis=1)
exploration_op = tf.random_uniform(tf.shape(logits))
```
Next, we'll define our memory and exploration strategies:
```python
# Define the memory and exploration strategies
replay_memory = []
def sample_memories(batch_size):
indices = np.random.permutation(len(replay_memory))[:batch_size]
cols = [[], [], [], [], []] # state, action, reward, next_state, done
for index in indices:
memory = replay_memory[index]
for col, value in zip(cols, memory):
col.append(value)
cols = [np.array(col) for col in cols]
return (cols[0], cols[1], cols[2].reshape(-1, 1), cols[3], cols[4].reshape(-1, 1))
epsilon = start_epsilon
def explore(state, step):
if step < epsilon_decay_steps:
epsilon = start_epsilon - step / epsilon_decay_steps * (start_epsilon - end_epsilon)
else:
epsilon = end_epsilon
if np.random.rand() < epsilon:
return np.random.randint(n_outputs)
else:
return predict_op.eval(feed_dict={X: state.reshape(1, n_inputs)})
```
Now we're ready to start training:
```python
# Start training
env = gym.make("CartPole-v0")
with tf.Session() as sess:
tf.global_variables_initializer().run()
for episode in range(n_episodes):
# Reset the environment
obs = env.reset()
for step in range(n_steps):
# Explore the environment
action = explore(obs, step)
next_obs, reward, done, _ = env.step(action)
# Store the experience in replay memory
replay_memory.append((obs, action, reward, next_obs, done))
obs = next_obs
# Sample a random batch of experiences from replay memory
X_state_val, X_action_val, rewards, X_next_state_val, dones = (sample_memories(batch_size))
# Compute the TD target
next_q_values = logits.eval(feed_dict={X: X_next_state_val})
max_next_q_values = np.max(next_q_values, axis=1, keepdims=True)
# Compute the training targets
y_val = rewards + (1 - dones) * gamma * max_next_q_values
# Train the network on a batch of experiences
training_op.run(feed_dict={X: X_state_val, y: y_val})
# Every 50 episodes, print the episode number and average reward
if episode % 50 == 0:
total_reward = 0
obs = env.reset()
for i in range(n_steps):
action = predict_op.eval(feed_dict={X: obs.reshape(1, n_inputs)})
obs, reward, done, _ = env.step(action)
total_reward += reward
if done:
break
print("Episode:", episode, " Reward:", total_reward)
```
And that's it! This program should train a neural network using the DQN method to solve the CartPole-V0 problem, and print the episode number and average reward every 50 episodes. Let me know if you have any questions or if there's anything else I can help you with!
dqn的python代码,用中午注解
很高兴为您解答这个问题。以下是 Deep Q-Network(DQN)的Python代码,带有中文注释说明:
```python
import gym
import numpy as np
import tensorflow as tf
from tensorflow import keras
# 设置超参数
ENV_NAME = "CartPole-v0"
MEMORY_SIZE = 100000 # 记忆池大小
BATCH_SIZE = 32 # 批量大小
GAMMA = 0.99 # 折扣因子
EPSILON_START = 1.0 # 初始探索率
EPSILON_MIN = 0.01 # 最低探索率
EPSILON_DECAY = 1000000 # 探索率衰减率
TARGET_UPDATE_FREQ = 1000 # 目标网络更新频率
# 创建环境
env = gym.make(ENV_NAME)
num_actions = env.action_space.n
# 创建Q网络和目标网络
q_net = keras.Sequential()
q_net.add(keras.layers.Dense(24, input_shape=env.observation_space.shape, activation="relu"))
q_net.add(keras.layers.Dense(24, activation="relu"))
q_net.add(keras.layers.Dense(num_actions, activation=None))
target_net = keras.models.clone_model(q_net)
target_net.set_weights(q_net.get_weights())
# 创建记忆池
memory_states = np.zeros((MEMORY_SIZE, env.observation_space.shape[0]))
memory_actions = np.zeros((MEMORY_SIZE,), dtype=np.uint8)
memory_rewards = np.zeros((MEMORY_SIZE,))
memory_next_states = np.zeros((MEMORY_SIZE, env.observation_space.shape[0]))
memory_dones = np.zeros((MEMORY_SIZE,), dtype=np.uint8)
memory_counter = 0
# 初始化探索率和步数计数器
epsilon = EPSILON_START
step_count = 0
# 定义损失函数和优化器
loss_func = keras.losses.mean_squared_error
optimizer = keras.optimizers.Adam(learning_rate=0.001)
# 训练Q网络
for episode in range(1000):
state = env.reset()
episode_reward = 0
done = False
while not done:
# 探索或利用
if np.random.rand() < epsilon:
action = env.action_space.sample()
else:
q_values = q_net.predict(np.expand_dims(state, axis=0))
action = np.argmax(q_values)
# 执行动作,得到下一个状态、奖励和是否结束标志
next_state, reward, done, _ = env.step(action)
episode_reward += reward
# 将记忆添加到记忆池中
memory_states[memory_counter] = state
memory_actions[memory_counter] = action
memory_rewards[memory_counter] = reward
memory_next_states[memory_counter] = next_state
memory_dones[memory_counter] = done
memory_counter = (memory_counter + 1) % MEMORY_SIZE
# 更新Q网络
if memory_counter >= BATCH_SIZE:
batch_indices = np.random.choice(MEMORY_SIZE, BATCH_SIZE, replace=False)
batch_states = memory_states[batch_indices]
batch_actions = memory_actions[batch_indices]
batch_rewards = memory_rewards[batch_indices]
batch_next_states = memory_next_states[batch_indices]
batch_dones = memory_dones[batch_indices]
q_values_next = target_net.predict_on_batch(batch_next_states)
max_q_values_next = np.max(q_values_next, axis=1)
targets = batch_rewards + (1 - batch_dones) * GAMMA * max_q_values_next
q_values = q_net.predict_on_batch(batch_states)
q_values[np.arange(BATCH_SIZE), batch_actions] = targets
loss = q_net.train_on_batch(batch_states, q_values)
# 更新目标网络
if step_count % TARGET_UPDATE_FREQ == 0:
target_net.set_weights(q_net.get_weights())
# 更新探索率和步数计数器
epsilon = max(EPSILON_MIN, EPSILON_START - step_count / EPSILON_DECAY)
step_count += 1
# 进入下一个状态
state = next_state
print(f"Episode {episode + 1}: reward = {episode_reward}")
```
这是一个简单的DQN实现,用于解决OpenAI Gym的CartPole环境。它使用TensorFlow 2.0构建神经网络,基于经验回放和固定Q目标的思想进行训练。在训练过程中,会逐渐降低探索率,提高利用率,从而使得智能体更加稳定地学习到正确的行为策略。
相关推荐
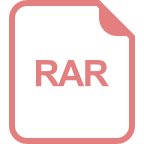
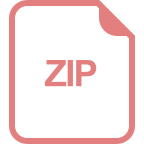
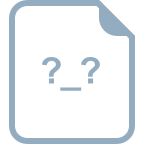








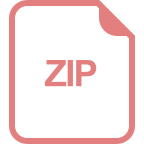
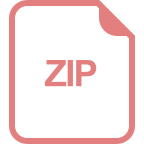
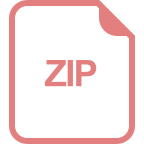
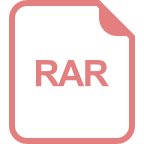