c语言中实现有以下字符串:****A*BC*DEF****,删除字符串中间的星号,得到如下结果:****ABCDEF****
时间: 2023-08-27 14:06:46 浏览: 68
可以使用C语言中的字符串处理函数来实现该功能,具体步骤如下:
1. 定义一个字符数组存储原始字符串,例如:
```c
char str[] = "****A*BC*DEF****";
```
2. 定义一个新的字符数组,用于存储去除星号后的字符串,例如:
```c
char new_str[13]; // 由于去除星号后的字符串长度为 10,需要额外占用 3 个字符的空间存储结尾的星号
```
3. 使用循环遍历原始字符串,将非星号字符逐个添加到新的字符数组中,例如:
```c
int j = 0; // 记录新数组的下标
for (int i = 0; i < strlen(str); i++) {
if (str[i] != '*') {
new_str[j++] = str[i];
}
}
```
4. 在新的字符数组的末尾添加结尾的星号,例如:
```c
new_str[j] = '*';
new_str[j+1] = '*';
new_str[j+2] = '*';
new_str[j+3] = '\0'; // 末尾添加字符串结束符
```
完整代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "****A*BC*DEF****";
char new_str[13];
int j = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] != '*') {
new_str[j++] = str[i];
}
}
new_str[j] = '*';
new_str[j+1] = '*';
new_str[j+2] = '*';
new_str[j+3] = '\0';
printf("%s", new_str);
return 0;
}
```
输出结果为:
```
****ABCDEF****
```
相关推荐
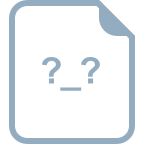
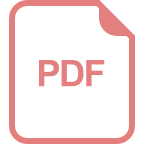
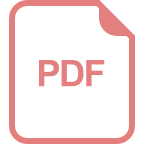













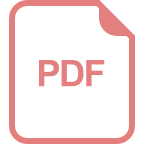