node.js+express+mysql实现博客系统代码
时间: 2023-08-06 07:03:31 浏览: 60
好的,以下是一个简单的示例代码,实现了一个基本的博客系统。
首先,我们需要创建一个新的node.js项目,并在项目中安装express和mysql模块。
```bash
mkdir blog
cd blog
npm init -y
npm install express mysql --save
```
然后,我们需要创建一个app.js文件,并在其中配置express应用程序。
```javascript
const express = require('express');
const mysql = require('mysql');
const app = express();
// 配置中间件
app.use(express.urlencoded({ extended: false }));
app.use(express.json());
app.use(express.static('public'));
// 连接数据库
const db = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'blog'
});
db.connect((err) => {
if (err) {
console.error('error connecting: ' + err.stack);
return;
}
console.log('connected as id ' + db.threadId);
});
// 设置路由
app.get('/', (req, res) => {
db.query('SELECT * FROM posts', (err, results) => {
if (err) throw err;
res.render('index.ejs', { posts: results });
});
});
app.get('/post/:id', (req, res) => {
const id = req.params.id;
db.query('SELECT * FROM posts WHERE id = ?', [id], (err, results) => {
if (err) throw err;
res.render('post.ejs', { post: results[0] });
});
});
app.get('/create', (req, res) => {
res.render('create.ejs');
});
app.post('/create', (req, res) => {
const title = req.body.title;
const content = req.body.content;
db.query('INSERT INTO posts SET ?', { title, content }, (err, results) => {
if (err) throw err;
res.redirect('/');
});
});
// 启动服务器
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
在上面的代码中,我们使用了ejs模板引擎来渲染HTML页面。我们需要在项目中创建一个views文件夹,并在其中创建index.ejs、post.ejs和create.ejs文件。
index.ejs:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Blog</title>
</head>
<body>
<h1>Blog</h1>
<ul>
<% for (let post of posts) { %>
<li>
<a href="/post/<%= post.id %>"><%= post.title %></a>
</li>
<% } %>
</ul>
<a href="/create">Create a new post</a>
</body>
</html>
```
post.ejs:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title><%= post.title %></title>
</head>
<body>
<h1><%= post.title %></h1>
<p><%= post.content %></p>
<a href="/">Back to index</a>
</body>
</html>
```
create.ejs:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Create a new post</title>
</head>
<body>
<h1>Create a new post</h1>
<form action="/create" method="POST">
<input type="text" name="title" placeholder="Title">
<textarea name="content" placeholder="Content"></textarea>
<button type="submit">Create</button>
</form>
</body>
</html>
```
最后,我们需要在项目中创建一个public文件夹,并在其中创建一个style.css文件,来为页面添加样式。
```css
body {
font-family: Arial, sans-serif;
}
h1 {
font-size: 32px;
font-weight: bold;
}
ul {
list-style: none;
padding: 0;
}
li {
margin-bottom: 10px;
}
a {
text-decoration: none;
color: blue;
}
input[type="text"],
textarea {
display: block;
width: 100%;
margin-bottom: 10px;
}
button[type="submit"] {
display: block;
margin-top: 10px;
}
```
以上就是一个简单的node.js+express+mysql实现博客系统的示例代码,可以根据实际情况进行调整和扩展。
相关推荐
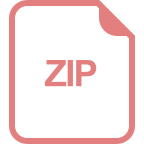
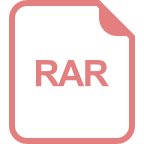














