学生选课系统c++
时间: 2023-07-29 19:07:11 浏览: 106
好的,学生选课系统是一个比较典型的管理信息系统,以下是一个简单的C++代码实现:
```c++
#include<iostream>
#include<fstream>
#include<cstdlib>
#include<iomanip>
using namespace std;
// 学生结构体
struct student{
int num; // 学号
string name; // 姓名
int age; // 年龄
int score; // 成绩
};
// 学生链表节点
struct node{
student data;
node *next;
};
node *head = NULL; // 头节点
int stu_num = 0; // 学生人数
// 显示菜单
void show_menu(){
cout << "===========================" << endl;
cout << " 学生选课系统 " << endl;
cout << "===========================" << endl;
cout << "1. 添加学生" << endl;
cout << "2. 删除学生" << endl;
cout << "3. 修改学生信息" << endl;
cout << "4. 查询学生信息" << endl;
cout << "5. 显示所有学生信息" << endl;
cout << "6. 保存学生信息到文件" << endl;
cout << "7. 从文件中读取学生信息" << endl;
cout << "0. 退出系统" << endl;
cout << "===========================" << endl;
}
// 添加学生
void add_student(){
student stu;
node *p = new node;
cout << "请输入学生学号:";
cin >> stu.num;
cout << "请输入学生姓名:";
cin >> stu.name;
cout << "请输入学生年龄:";
cin >> stu.age;
cout << "请输入学生成绩:";
cin >> stu.score;
p->data = stu;
p->next = head;
head = p;
stu_num++;
cout << "学生添加成功!" << endl;
}
// 删除学生
void del_student(){
int num;
node *p = head, *q = head;
cout << "请输入要删除的学生学号:";
cin >> num;
while(p != NULL){
if(p->data.num == num){
if(p == head){
head = p->next;
}else{
q->next = p->next;
}
delete p;
stu_num--;
cout << "学生删除成功!" << endl;
return;
}
q = p;
p = p->next;
}
cout << "学生不存在!" << endl;
}
// 修改学生信息
void modify_student(){
int num;
node *p = head;
cout << "请输入要修改的学生学号:";
cin >> num;
while(p != NULL){
if(p->data.num == num){
cout << "请输入学生姓名:";
cin >> p->data.name;
cout << "请输入学生年龄:";
cin >> p->data.age;
cout << "请输入学生成绩:";
cin >> p->data.score;
cout << "学生信息修改成功!" << endl;
return;
}
p = p->next;
}
cout << "学生不存在!" << endl;
}
// 查询学生信息
void query_student(){
int num;
node *p = head;
cout << "请输入要查询的学生学号:";
cin >> num;
while(p != NULL){
if(p->data.num == num){
cout << setw(10) << "学号" << setw(10) << "姓名" << setw(10) << "年龄" << setw(10) << "成绩" << endl;
cout << setw(10) << p->data.num << setw(10) << p->data.name << setw(10) << p->data.age << setw(10) << p->data.score << endl;
return;
}
p = p->next;
}
cout << "学生不存在!" << endl;
}
// 显示所有学生信息
void show_all_student(){
node *p = head;
cout << setw(10) << "学号" << setw(10) << "姓名" << setw(10) << "年龄" << setw(10) << "成绩" << endl;
while(p != NULL){
cout << setw(10) << p->data.num << setw(10) << p->data.name << setw(10) << p->data.age << setw(10) << p->data.score << endl;
p = p->next;
}
}
// 保存学生信息到文件
void save_to_file(){
ofstream outfile("students.txt", ios::out);
if(!outfile){
cerr << "文件打开失败!" << endl;
exit(1);
}
node *p = head;
while(p != NULL){
outfile << p->data.num << " " << p->data.name << " " << p->data.age << " " << p->data.score << endl;
p = p->next;
}
outfile.close();
cout << "学生信息保存成功!" << endl;
}
// 从文件中读取学生信息
void read_from_file(){
ifstream infile("students.txt", ios::in);
if(!infile){
cerr << "文件打开失败!" << endl;
exit(1);
}
student stu;
while(infile >> stu.num >> stu.name >> stu.age >> stu.score){
node *p = new node;
p->data = stu;
p->next = head;
head = p;
stu_num++;
}
infile.close();
cout << "学生信息读取成功!" << endl;
}
int main(){
int choice;
while(true){
show_menu();
cout << "请输入您的选择:";
cin >> choice;
switch(choice){
case 0: // 退出系统
exit(0);
case 1: // 添加学生
add_student();
break;
case 2: // 删除学生
del_student();
break;
case 3: // 修改学生信息
modify_student();
break;
case 4: // 查询学生信息
query_student();
break;
case 5: // 显示所有学生信息
show_all_student();
break;
case 6: // 保存学生信息到文件
save_to_file();
break;
case 7: // 从文件中读取学生信息
read_from_file();
break;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
这个学生选课系统实现了基本的增删改查功能,可以通过菜单选择实现不同的功能。同时,还支持将学生信息保存到文件和从文件中读取学生信息。当然,这只是一个简单的实现,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐




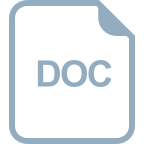
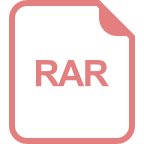









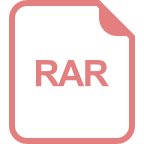

