python对文本进行词频分析,并用excel统计
时间: 2023-07-05 17:27:07 浏览: 140
好的,用Python进行文本词频分析可以使用NLTK库。首先需要安装NLTK库,安装命令如下:
```
pip install nltk
```
然后,可以按照以下步骤进行文本词频分析:
1. 导入所需库
```python
import nltk
from nltk import FreqDist
from nltk.corpus import stopwords
import pandas as pd
```
2. 读取文本文件并进行分词
```python
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
tokens = nltk.word_tokenize(text)
```
3. 去除停用词和标点符号
```python
stop_words = set(stopwords.words('english'))
filtered_tokens = [token.lower() for token in tokens if token.isalpha() and token.lower() not in stop_words]
```
4. 统计词频并输出前10个高频词
```python
freq_dist = FreqDist(filtered_tokens)
print(freq_dist.most_common(10))
```
5. 导出词频统计结果到Excel
```python
df = pd.DataFrame(freq_dist.items(), columns=['Word', 'Frequency'])
df.to_excel('freq_dist.xlsx', index=False)
```
以上就是Python进行文本词频分析并用Excel统计的步骤。
相关推荐
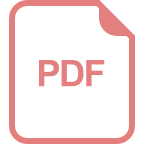
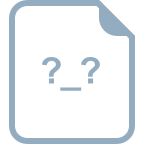














