请用C语言编写如下程序:请用指针计算两个矩阵的和
时间: 2023-06-16 22:06:26 浏览: 54
以下是用指针计算两个矩阵的和的C语言程序:
```c
#include <stdio.h>
void add_matrices(int *m1, int *m2, int *result, int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
*(result + i * cols + j) = *(m1 + i * cols + j) + *(m2 + i * cols + j);
}
}
}
void print_matrix(int *matrix, int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", *(matrix + i * cols + j));
}
printf("\n");
}
}
int main() {
int rows, cols;
printf("Enter the number of rows and columns of matrices: ");
scanf("%d %d", &rows, &cols);
int m1[rows][cols], m2[rows][cols], result[rows][cols];
printf("Enter the elements of first matrix:\n");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
scanf("%d", &m1[i][j]);
}
}
printf("Enter the elements of second matrix:\n");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
scanf("%d", &m2[i][j]);
}
}
add_matrices(&m1[0][0], &m2[0][0], &result[0][0], rows, cols);
printf("Resultant matrix is:\n");
print_matrix(&result[0][0], rows, cols);
return 0;
}
```
在这个程序中,我们首先定义了一个 `add_matrices()` 函数,它使用指针来计算两个矩阵的和。然后,我们定义了一个 `print_matrix()` 函数来打印矩阵。在 `main()` 函数中,我们首先获取矩阵的行数和列数。然后,我们使用两个嵌套的循环来获取两个矩阵的元素。接下来,我们调用 `add_matrices()` 函数来计算两个矩阵的和,并将结果存储在 `result` 数组中。最后,我们使用 `print_matrix()` 函数来打印结果矩阵。
请注意,在 `add_matrices()` 和 `print_matrix()` 函数中,我们使用了指针算术来访问矩阵的元素。特别地,我们使用了 `*(matrix + i * cols + j)` 的形式来访问第 `i` 行第 `j` 列的元素,其中 `matrix` 是指向矩阵的指针,`cols` 是矩阵的列数。
相关推荐
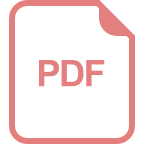
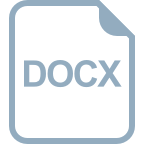
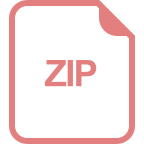















