jtable 合并单元格 详细代码
时间: 2024-05-02 08:04:09 浏览: 139
以下是使用 JTable 实现单元格合并的详细代码:
首先,我们需要自定义一个 TableModel 类来实现单元格合并:
```
import javax.swing.table.AbstractTableModel;
import java.util.HashMap;
import java.util.Map;
public class MergeTableModel extends AbstractTableModel {
private Object[][] data;
private String[] columnNames;
private Map<CellIndex, CellSpan> spanMap;
public MergeTableModel(Object[][] data, String[] columnNames) {
this.data = data;
this.columnNames = columnNames;
this.spanMap = new HashMap<>();
}
public void merge(int startRow, int endRow, int startCol, int endCol) {
spanMap.put(new CellIndex(startRow, startCol), new CellSpan(endRow - startRow + 1, endCol - startCol + 1));
}
@Override
public int getRowCount() {
return data.length;
}
@Override
public int getColumnCount() {
return columnNames.length;
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
CellSpan span = spanMap.get(new CellIndex(rowIndex, columnIndex));
if (span != null) {
return null;
}
return data[rowIndex][columnIndex];
}
@Override
public boolean isCellEditable(int rowIndex, int columnIndex) {
return false;
}
public int getRealRow(int row) {
int realRow = row;
for (CellIndex cellIndex : spanMap.keySet()) {
CellSpan span = spanMap.get(cellIndex);
int endRow = cellIndex.row + span.row - 1;
if (row >= cellIndex.row && row <= endRow) {
realRow = cellIndex.row;
break;
}
}
return realRow;
}
public int getRealColumn(int column) {
int realColumn = column;
for (CellIndex cellIndex : spanMap.keySet()) {
CellSpan span = spanMap.get(cellIndex);
int endColumn = cellIndex.column + span.column - 1;
if (column >= cellIndex.column && column <= endColumn) {
realColumn = cellIndex.column;
break;
}
}
return realColumn;
}
public int getRowSpan(int row, int column) {
CellSpan span = spanMap.get(new CellIndex(row, column));
if (span != null) {
return span.row;
}
return 1;
}
public int getColumnSpan(int row, int column) {
CellSpan span = spanMap.get(new CellIndex(row, column));
if (span != null) {
return span.column;
}
return 1;
}
private static class CellIndex {
private int row;
private int column;
private CellIndex(int row, int column) {
this.row = row;
this.column = column;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CellIndex cellIndex = (CellIndex) o;
if (row != cellIndex.row) return false;
return column == cellIndex.column;
}
@Override
public int hashCode() {
int result = row;
result = 31 * result + column;
return result;
}
}
private static class CellSpan {
private int row;
private int column;
private CellSpan(int row, int column) {
this.row = row;
this.column = column;
}
}
}
```
然后,我们在创建 JTable 的时候使用自定义的 TableModel 类,并在需要合并单元格的地方调用 merge 方法:
```
// 创建数据和列名
Object[][] data = {
{"1", "A", "B", "C"},
{"2", "D", "E", "F"},
{"3", "G", "H", "I"},
{"4", "J", "K", "L"}
};
String[] columnNames = {"#", "Column 1", "Column 2", "Column 3"};
// 创建自定义 TableModel
MergeTableModel tableModel = new MergeTableModel(data, columnNames);
// 创建 JTable
JTable table = new JTable(tableModel);
// 合并单元格
tableModel.merge(0, 1, 1, 2);
tableModel.merge(2, 3, 2, 3);
// 创建 JScrollPane 并将 JTable 添加到其中
JScrollPane scrollPane = new JScrollPane(table);
```
最后,我们需要重写 JTable 的 getCellRenderer 方法来实现单元格的合并显示:
```
// 创建 DefaultTableCellRenderer
DefaultTableCellRenderer renderer = new DefaultTableCellRenderer() {
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
Component c = super.getTableCellRendererComponent(table, value, isSelected, hasFocus, row, column);
int realRow = tableModel.getRealRow(row);
int realColumn = tableModel.getRealColumn(column);
int rowSpan = tableModel.getRowSpan(realRow, realColumn);
int colSpan = tableModel.getColumnSpan(realRow, realColumn);
if (rowSpan > 1 || colSpan > 1) {
Rectangle rect = table.getCellRect(realRow, realColumn, false);
for (int i = realRow; i < realRow + rowSpan; i++) {
for (int j = realColumn; j < realColumn + colSpan; j++) {
if (i == realRow && j == realColumn) {
continue;
}
rect.add(table.getCellRect(i, j, false));
}
}
c.setBounds(rect);
((JComponent) c).setOpaque(false);
} else {
((JComponent) c).setOpaque(true);
}
return c;
}
};
// 设置渲染器
table.setDefaultRenderer(Object.class, renderer);
```
阅读全文
相关推荐



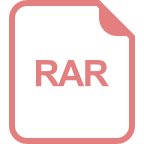










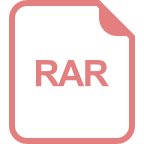
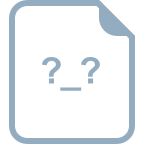


