torch.tensor与pil.image转换代码
时间: 2023-12-01 10:04:35 浏览: 135
Sure, here is an example code snippet for converting a PIL Image to a PyTorch tensor and vice versa:
```
import torch
import torchvision.transforms as transforms
from PIL import Image
# PIL Image to PyTorch tensor
pil_image = Image.open('image.jpg')
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
tensor_image = transform(pil_image)
# PyTorch tensor to PIL Image
tensor_image = torch.randn(3, 224, 224)
tensor_image = ((tensor_image + 1) / 2) * 255 # un-normalize
pil_image = transforms.ToPILImage()(tensor_image)
```
Hope this helps!
相关问题
torch.tensor可以转换PIL对象吗
是的,可以使用Pillow(PIL)库将PIL图像转换为PyTorch张量,也可以将PyTorch张量转换为PIL图像。下面是一个示例:
```python
from PIL import Image
import torch
# 转换PIL图像为PyTorch张量
pil_image = Image.open('image.jpg')
tensor_image = torch.tensor(pil_image)
# 转换PyTorch张量为PIL图像
tensor_image = torch.randn(3, 256, 256) # 生成一个随机的3通道256x256张量
pil_image = Image.fromarray(tensor_image.numpy())
```
需要注意的是,PIL图像和PyTorch张量的形状和数据类型可能会有所不同,需要根据具体情况进行转换。
torch.tensor和transform.totensor
torch.tensor和transform.totensor都是PyTorch库中用来处理张量数据的函数。
torch.tensor是PyTorch中创建张量的函数之一。它可以将一个Python列表、元组、NumPy数组等转换为PyTorch张量对象。例如,可以使用torch.tensor将一个Python列表转换为张量:
```python
import torch
data = [1, 2, 3, 4, 5]
tensor_data = torch.tensor(data)
```
transform.totensor是在torchvision库中的一个函数,用于将PIL图像或NumPy数组转换为PyTorch张量。这在计算机视觉任务中非常常见,因为在进行深度学习模型训练时,图像数据通常需要以张量的形式输入模型。
```python
from torchvision import transforms
# 将PIL图像转换为张量
image = Image.open('image.jpg')
tensor_image = transforms.ToTensor()(image)
# 将NumPy数组转换为张量
numpy_array = np.array([[1, 2, 3], [4, 5, 6]])
tensor_array = transforms.ToTensor()(numpy_array)
```
总结起来,torch.tensor适用于一般的张量创建,而transform.totensor适用于将图像或数组转换为张量。
阅读全文
相关推荐
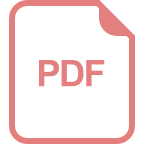
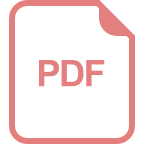
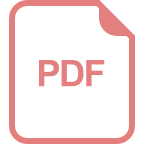













