pytorch一个编码器两个解码器模型
时间: 2023-08-09 21:09:24 浏览: 62
下面是一个使用PyTorch实现的一个编码器和两个解码器的模型:
```python
import torch
import torch.nn as nn
class EncoderDecoder(nn.Module):
def __init__(self):
super(EncoderDecoder, self).__init__()
self.encoder_fc1 = nn.Linear(in_features=784, out_features=256)
self.encoder_fc2 = nn.Linear(in_features=256, out_features=64)
self.encoder_fc3 = nn.Linear(in_features=64, out_features=16)
self.decoder_fc1 = nn.Linear(in_features=16, out_features=64)
self.decoder_fc2 = nn.Linear(in_features=64, out_features=256)
self.decoder_fc3 = nn.Linear(in_features=256, out_features=784)
self.decoder_fc4 = nn.Linear(in_features=256, out_features=10)
def encode(self, x):
x = torch.relu(self.encoder_fc1(x))
x = torch.relu(self.encoder_fc2(x))
x = torch.relu(self.encoder_fc3(x))
return x
def decode1(self, x):
x = torch.relu(self.decoder_fc1(x))
x = torch.relu(self.decoder_fc2(x))
x = torch.sigmoid(self.decoder_fc3(x))
return x
def decode2(self, x):
x = torch.relu(self.decoder_fc1(x))
x = torch.relu(self.decoder_fc2(x))
x = self.decoder_fc4(x)
return x
def forward(self, x):
x = self.encode(x)
out1 = self.decode1(x)
out2 = self.decode2(x)
return out1, out2
```
这个模型包含一个编码器和两个解码器。编码器包含三个全连接层,将输入的784维向量压缩到16维。两个解码器都包含四个全连接层,其中前三个层的结构与编码器相同,最后一层的输出维度分别是784和10,对应于重构图像和分类标签。模型的前向传播过程首先将输入数据通过编码器进行编码,然后分别通过两个解码器进行解码,得到重构图像和分类标签的预测结果。
相关推荐
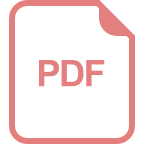
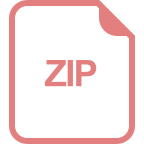
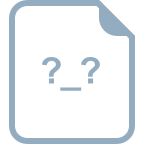
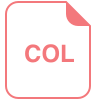
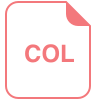











