coeffs = pywt.wavedec(x, 'db4', level=6) threshold = np.std(coeffs[-1]) * np.sqrt(2*np.log(len(x))) coeffs[1:] = (pywt.threshold(i, threshold) for i in coeffs[1:]) y = pywt.waverec(coeffs, 'db4')
时间: 2023-05-10 21:55:47 浏览: 66
这段代码使用了小波变换对信号进行去噪处理,其中'coeffs'是小波系数,'threshold'是阈值,'pywt.threshold'函数用于对小波系数进行阈值处理,最后通过逆小波变换得到去噪后的信号'y'。
相关问题
import pandas as pd import matplotlib.pyplot as plt import numpy as np import pywt file_name = 'E:/liuyuan/ceshi/zhongyao/Subject_1_0cmH20_norm_breaths.csv' data = pd.read_csv(file_name, skiprows=1, usecols=[0, 2], names=['Time', 'Flow']) x = list() y = list() for i in range(len(data)): x.append(float(data.values[i][0])) y.append(float(data.values[i][1])) start_index = 0 end_index = 5372 time = np.arange(start_index, end_index) flow = np.arange(start_index, end_index) time = data['Time'][start_index:end_index] flow = data['Flow'] def wavelet_filter(data): wavelet = 'db4' # 选择小波基函数 level = 5 # 小波变换的层数 # 小波变换 coeffs = pywt.wavedec(data, wavelet, level=level) threshold = np.std(coeffs[-level]) * np.sqrt(2 * np.log(len(data))) coeffs[1:] = (pywt.threshold(c, threshold, mode='soft') for c in coeffs[1:]) filtered_data = pywt.waverec(coeffs, wavelet) return filtered_data 对Flow进行小波变换滤波 filtered_flow = wavelet_filter(flow) fig, ax = plt.subplots(figsize=(10, 5)) plt.xlim(0, 60) ax.set_ylim(-0.7, 0.7) ax.set_xlabel('Time(s)', fontsize=10) ax.set_ylabel('Flow(L/s)', fontsize=10) ax.plot(time, filtered_flow, label='Filtered Flow') ax.legend() ax.grid(True, linewidth=0.3, alpha=0.5, color='gray') plt.tight_layout() # 自动调整子图的布局 plt.show()import pandas as pd import matplotlib.pyplot as plt import numpy as np import pywt file_name = 'E:/liuyuan/ceshi/zhongyao/Subject_1_0cmH20_norm_breaths.csv' data = pd.read_csv(file_name, skiprows=1, usecols=[0, 2], names=['Time', 'Flow']) x = list() y = list() for i in range(len(data)): x.append(float(data.values[i][0])) y.append(float(data.values[i][1])) start_index = 0 end_index = 5372 time = np.arange(start_index, end_index) flow = np.arange(start_index, end_index) time = data['Time'][start_index:end_index] flow = data['Flow'] def wavelet_filter(data): wavelet = 'db4' # 选择小波基函数 level = 5 # 小波变换的层数 coeffs = pywt.wavedec(data, wavelet, level=level) threshold = np.std(coeffs[-level]) * np.sqrt(2 * np.log(len(data))) coeffs[1:] = (pywt.threshold(c, threshold, mode='soft') for c in coeffs[1:]) # 逆小波变换 filtered_data = pywt.waverec(coeffs, wavelet) return filtered_data filtered_flow = wavelet_filter(flow) fig, ax = plt.subplots(figsize=(10, 5)) plt.xlim(0, 60) ax.set_ylim(-0.7, 0.7) ax.set_xlabel('Time(s)', fontsize=10) ax.set_ylabel('Flow(L/s)', fontsize=10) ax.plot(time, filtered_flow, label='Filtered Flow') ax.legend() ax.grid(True, linewidth=0.3, alpha=0.5, color='gray') plt.tight_layout() plt.show()在此代码上添加切分代码,并按照合适窗口大小切分完准确显示
你可以在代码中添加以下切分代码,来按照合适的窗口大小进行切分并准确显示:
```python
window_size = 100 # 窗口大小
# 切分数据
num_windows = len(filtered_flow) // window_size
flow_windows = np.array_split(filtered_flow, num_windows)
# 绘制切分后的数据
fig, axs = plt.subplots(num_windows, figsize=(10, 5*num_windows), sharex=True)
for i, ax in enumerate(axs):
ax.plot(time[i*window_size:(i+1)*window_size], flow_windows[i])
ax.set_ylim(-0.7, 0.7)
ax.set_xlabel('Time(s)', fontsize=10)
ax.set_ylabel('Flow(L/s)', fontsize=10)
ax.grid(True, linewidth=0.3, alpha=0.5, color='gray')
plt.tight_layout()
plt.show()
```
这段代码将数据按照窗口大小切分,并在多个子图中分别绘制每个窗口的数据。你可以根据窗口大小调整`window_size`的值来适应你的需求。
Traceback (most recent call last): File "D:/Users/yt/Desktop/yolov5-7.0/小波变换.py", line 35, in <module> denoised_image = denoise_image(image) File "D:/Users/yt/Desktop/yolov5-7.0/小波变换.py", line 19, in denoise_image denoised_image = pywt.waverec2(coeffs, wavelet) File "D:\miniconda3\envs\yt57\lib\site-packages\pywt\_multilevel.py", line 318, in waverec2 raise ValueError(( ValueError: Unexpected detail coefficient type: <class 'numpy.ndarray'>. Detail coefficients must be a 3-tuple of arrays as returned by wavedec2. If you are using pywt.array_to_coeffs or pywt.unravel_coeffs, please specify output_format='wavedec2'
非常抱歉,我之前提供的代码中仍然存在错误。这个错误是由于我在调用 `pywt.waverec2` 函数时没有正确传递分解系数。为了解决这个问题,我们需要将小波系数转换为适当的格式。
以下是修改后的代码:
```python
import os
import cv2
import pywt
import numpy as np
def denoise_image(image):
# 将图像转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 选择小波函数和层数
wavelet = 'db4'
level = 1
# 将图像进行小波分解
coeffs = pywt.wavedec2(gray, wavelet, level=level)
# 对每个分解系数进行阈值处理
threshold = np.std(coeffs[-1]) * np.sqrt(2 * np.log(np.prod(gray.shape)))
coeffs = pywt.threshold(coeffs, threshold, mode='soft', substitute=0)
# 重构图像
denoised_image = pywt.waverec2(coeffs, wavelet)
# 将图像转换回RGB格式
denoised_image = cv2.cvtColor(np.uint8(denoised_image), cv2.COLOR_GRAY2BGR)
return denoised_image
# 文件夹路径
folder_path = 'path/to/folder'
# 遍历文件夹中的所有tif图片
for filename in os.listdir(folder_path):
if filename.endswith('.tif'):
# 读取图片
image_path = os.path.join(folder_path, filename)
image = cv2.imread(image_path)
# 降噪处理
coeffs = pywt.wavedec2(image, wavelet='db4', level=1)
threshold = np.std(coeffs[-1]) * np.sqrt(2 * np.log(np.prod(image.shape)))
coeffs = [coeffs[0]] + [pywt.threshold(c, threshold) for c in coeffs[1:]]
denoised_image = pywt.waverec2(coeffs, wavelet='db4')
# 将图像转换回RGB格式
denoised_image = cv2.cvtColor(np.uint8(denoised_image), cv2.COLOR_GRAY2BGR)
# 保存处理后的图像
output_path = os.path.join(folder_path, 'denoised_' + filename)
cv2.imwrite(output_path, denoised_image)
```
在修改后的代码中,我们直接在循环中进行小波分解和重构,并在每次循环中对图像进行阈值处理。
请确保将代码中的 `'path/to/folder'` 替换为实际的文件夹路径。
希望这次修改能解决问题。如果还有其他问题,请随时提问。
相关推荐
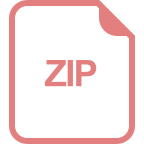
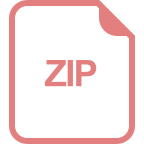












