iris.csv文件:4个特征数据,1个标签数据(0,1,2表示)。 使用pandas读取iris.csv文件,然后作为一个数据库表"iristab"写入MySQL数据库"studentdb"中。 从MySQL数据库studentdb中读出iris数据,把保存为一个Excel文件。
时间: 2024-02-13 09:07:58 浏览: 75
好的,我会为你提供如何完成这个任务的一般步骤。请注意,以下是一个基本的指南,你需要进行一些必要的修改以适应你的具体情况。
1. 导入必要的库和模块。
```python
import pandas as pd
import mysql.connector as mysql
from sqlalchemy import create_engine
```
2. 读取 iris.csv 文件,并将其存储为 pandas 数据帧。
```python
iris_df = pd.read_csv("iris.csv")
```
3. 连接 MySQL 数据库,并创建一个名为 "iristab" 的表。
```python
db = mysql.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="studentdb"
)
cursor = db.cursor()
# 创建表
cursor.execute("CREATE TABLE iristab (sepal_length FLOAT, sepal_width FLOAT, petal_length FLOAT, petal_width FLOAT, label INT)")
```
4. 将 iris 数据帧中的每一行插入到 MySQL 数据库的 "iristab" 表中。
```python
# 将数据插入表中
for i, row in iris_df.iterrows():
sql_query = f"INSERT INTO iristab VALUES ({row['sepal_length']}, {row['sepal_width']}, {row['petal_length']}, {row['petal_width']}, {row['label']})"
cursor.execute(sql_query)
db.commit()
```
5. 从 MySQL 数据库中读取 "iristab" 表,并将其存储为 pandas 数据帧。
```python
# 从表中检索数据
db_engine = create_engine('mysql+mysqlconnector://yourusername:yourpassword@localhost:3306/studentdb')
iristab_df = pd.read_sql_table('iristab', db_engine)
```
6. 将数据帧保存为 Excel 文件。
```python
# 将数据帧保存为 Excel 文件
iristab_df.to_excel("iris_data.xlsx", index=False)
```
这就是完成这个任务的大致步骤。请注意,在实际情况中,你需要根据你的具体情况进行适当的更改。
阅读全文
相关推荐
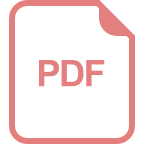













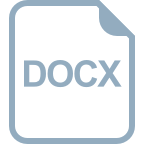