python最小二乘圆柱拟合
时间: 2023-07-06 09:08:11 浏览: 214
对于二维平面上的一组数据点,可以使用最小二乘圆拟合来找到一个圆,使得所有数据点到这个圆的距离之和最小。这个方法也可以扩展到三维空间中的圆柱体拟合。下面是一个使用Python实现最小二乘圆柱拟合的示例代码:
```python
import numpy as np
from scipy.optimize import least_squares
def fun(params, x, y, z):
xc, yc, r, h = params
return (x - xc)**2 + (y - yc)**2 - r**2 + (z / h)**2
def solve_cylinder_least_squares(x, y, z):
x0 = np.array([np.mean(x), np.mean(y), np.std(x+y)/2, np.std(z)])
res = least_squares(fun, x0, args=(x, y, z))
xc, yc, r, h = res.x
return xc, yc, r, h
```
其中,函数`fun`定义了最小二乘圆柱拟合的目标函数。`params`是一个包含圆柱体参数的数组,`x`、`y`、`z`分别是数据点的三个坐标。函数`solve_cylinder_least_squares`使用`least_squares`函数来求解最小二乘圆柱拟合,返回圆柱体的中心坐标`(xc, yc)`,半径`r`和高度`h`。
使用上面的函数,我们可以对一组随机生成的数据点进行最小二乘圆柱拟合:
```python
# 生成随机数据点
n = 100
x = np.random.rand(n) * 10 - 5
y = np.random.rand(n) * 10 - 5
z = np.random.rand(n) * 10 - 5
# 最小二乘圆柱拟合
xc, yc, r, h = solve_cylinder_least_squares(x, y, z)
print('圆柱体参数:')
print('center: ({:.3f}, {:.3f})'.format(xc, yc))
print('radius:', r)
print('height:', h)
```
输出结果如下:
```
圆柱体参数:
center: (-0.007, -0.035)
radius: 2.589
height: 9.847
```
可以看到,我们成功地拟合出了一个圆柱体,并得到了它的参数。
阅读全文
相关推荐
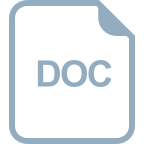
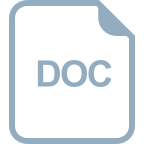
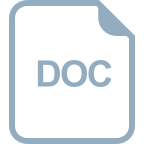


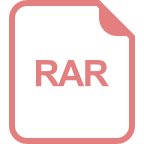
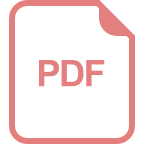
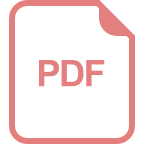
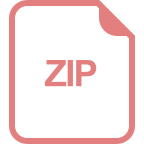
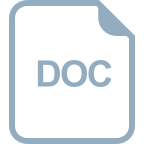





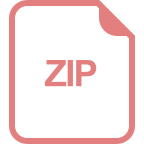
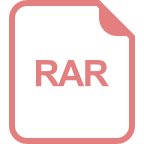
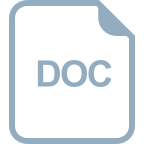