编写一段python代码将Mysql中的数据可视化到web页面
时间: 2023-09-21 16:05:52 浏览: 140
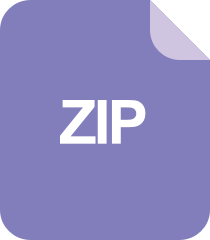
python制作Web可视化页面

### 回答1:
import pymysql
import pandas as pd
# 连接数据库
db = pymysql.connect(host="localhost",user="username",password="password",db="database")
# 获取游标
cursor = db.cursor()
# 执行SQL查询
cursor.execute("SELECT * FROM table")
# 获取查询结果
data = cursor.fetchall()
# 关闭数据库
db.close()
# 将查询结果以DataFrame格式存储
df = pd.DataFrame(list(data))
# 将DataFrame转化为html文件
html = df.to_html()
# 将html文件保存到web页面
with open('data.html','w') as f:
f.write(html)
### 回答2:
编写Python代码实现将MySQL中的数据可视化到Web页面可以使用Python的Flask框架和Matplotlib库来实现。
首先,需要安装Flask和Matplotlib库以及相关的依赖项。使用以下命令安装它们:
```
pip install flask
pip install matplotlib
```
接下来,可以编写一个Flask应用程序,用于连接MySQL数据库并获取数据。示例代码如下:
```python
from flask import Flask, render_template
import matplotlib.pyplot as plt
import mysql.connector
app = Flask(__name__)
@app.route('/')
def index():
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 获取数据
cursor = mydb.cursor()
cursor.execute("SELECT * FROM yourtable")
data = cursor.fetchall()
# 生成柱状图
x = [row[0] for row in data]
y = [row[1] for row in data]
fig, ax = plt.subplots()
ax.bar(x, y)
# 将图表保存为临时文件
chart_path = "/path/to/chart.png"
plt.savefig(chart_path)
return render_template('index.html', chart_path=chart_path)
if __name__ == '__main__':
app.run()
```
在上面的代码中,需要将`yourusername`、`yourpassword`、`yourdatabase`和`yourtable`替换为实际的MySQL数据库连接信息和表名。
接下来,需要创建一个名为`index.html`的模板文件,用于显示数据可视化图表。示例代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>Data Visualization</title>
</head>
<body>
<h1>Data Visualization</h1>
<img src="{{ chart_path }}" alt="Chart">
</body>
</html>
```
在上面的代码中,使用了Flask的模板引擎来渲染模板文件,并将图表的路径传递给模板文件中的`chart_path`变量。
最后,运行Flask应用程序,可以在浏览器中访问`http://localhost:5000`来查看数据可视化图表。
以上就是用Python编写实现将MySQL中的数据可视化到Web页面的简单示例代码。实际使用中,还可以根据需求进行进一步的定制和优化。
### 回答3:
要编写一段Python代码将MySQL中的数据可视化到web页面,需使用以下步骤:
1. 导入所需的库:需要包含MySQL连接库和web框架库,如`pymysql`和`flask`。
```python
import pymysql
from flask import Flask, render_template
```
2. 连接到MySQL数据库:使用`pymysql`库连接到MySQL数据库,并获取游标对象。
```python
conn = pymysql.connect(host='localhost', user='root', password='password', db='database_name')
cursor = conn.cursor()
```
3. 查询MySQL数据库的数据:使用游标对象执行SQL查询语句,获取需要的数据。
```python
sql = "SELECT * FROM table_name"
cursor.execute(sql)
data = cursor.fetchall()
```
4. 使用web框架创建一个简单的web应用程序:使用`flask`库创建一个简单的应用程序,并设置路由。
```python
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html', data=data)
if __name__ == '__main__':
app.run()
```
5. 创建一个HTML模板文件:在项目的根目录下创建一个名为`index.html`的文件,用于展示数据。
```html
<!DOCTYPE html>
<html>
<head>
<title>MySQL数据可视化</title>
</head>
<body>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
{% for row in data %}
<tr>
<td>{{ row[0] }}</td>
<td>{{ row[1] }}</td>
<td>{{ row[2] }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
6. 运行应用程序:在命令行中运行Python脚本,然后访问`http://localhost:5000`即可在web页面上看到MySQL数据库中的数据。
以上是一个简单的示例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
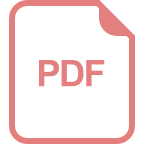



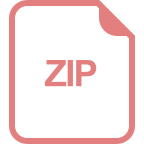
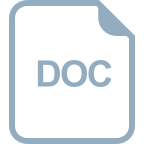
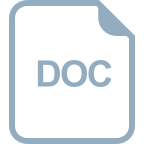
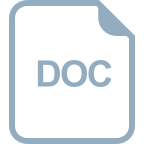
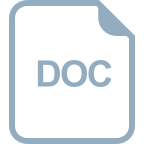
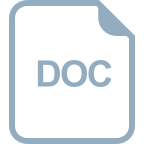
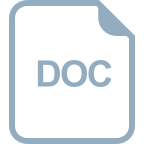
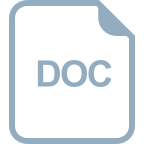
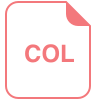
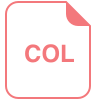
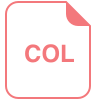
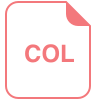
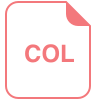