Python图形化网络数据可视化:拓扑工具使用手册
发布时间: 2024-09-11 16:06:44 阅读量: 196 订阅数: 74 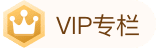
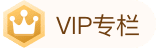
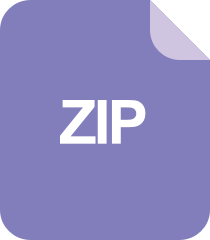
PSSE用户手册.zip_PSSE_PSSE用户手册_pss/e_se用户手册

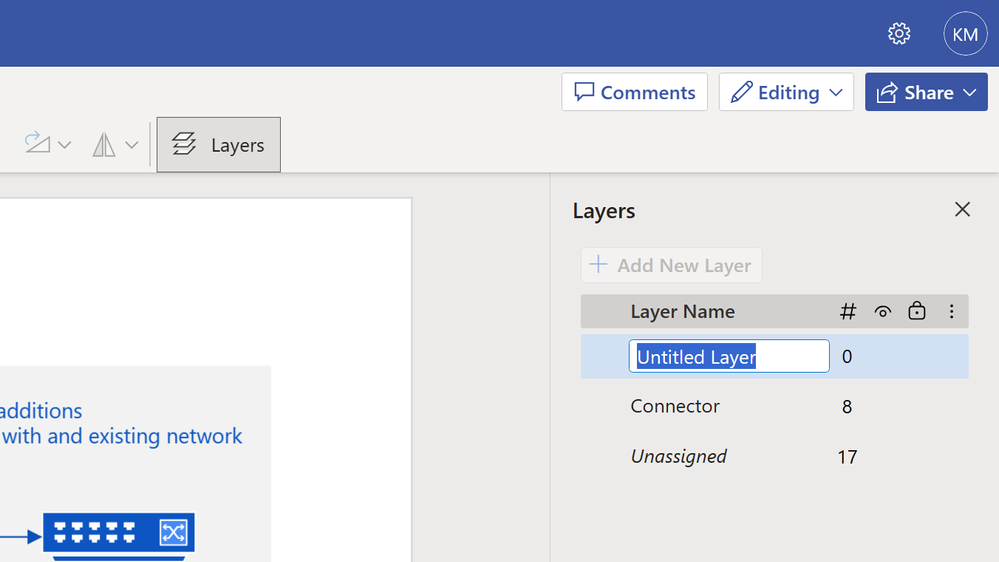
# 1. Python图形化网络数据可视化的概念和作用
## 1.1 图形化网络数据可视化的概述
在信息技术不断进步的今天,数据可视化已成为一个重要的研究和应用领域。图形化网络数据可视化是将抽象的网络数据以直观、形象的方式展现出来,帮助用户快速理解数据所蕴含的信息。Python,作为一种广泛使用的编程语言,提供了一系列图形化工具,如Matplotlib、Seaborn等,这些工具可以将复杂的数据转换为易于理解的图表和图形,大大提高了数据分析的效率。
## 1.2 图形化网络数据可视化的重要性
网络数据往往具有高度的复杂性和动态性,传统的数据分析方法很难直观展现其内在的模式和关联。图形化网络数据可视化技术的作用在于,它不仅能够揭示数据的结构,还可以辅助发现数据中的异常、趋势和相关性,为网络管理和安全决策提供科学依据。此外,它在提升用户体验、辅助科研发现和商业决策等方面都起到了不可替代的作用。
## 1.3 Python在图形化网络数据可视化中的优势
Python在图形化网络数据可视化方面的优势主要体现在其强大的社区支持和丰富的第三方库。这些库不仅功能全面,涵盖了数据处理、图表绘制和交互式可视化等多个方面,还能够轻松地与其他系统和库进行集成。另外,Python简洁易学的语法和广泛的应用场景使其成为数据科学家和开发者的首选。通过使用Python进行网络数据可视化,用户能够以较低的学习成本实现高效的可视化解决方案。
# 2. Python图形化工具的理论基础
### 2.1 Python图形化工具的基本语法和命令
#### 2.1.1 Python图形化工具的基本语法
Python作为一门强大的编程语言,在其众多库中,Tkinter库是实现图形化用户界面(GUI)的首选工具之一。Tkinter拥有丰富的小部件(widgets),例如按钮、标签、输入框等,它们可以通过简单的语法组合成复杂的用户界面。
下面是一个简单的Tkinter程序示例:
```python
import tkinter as tk
def say_hello():
print("Hello, world!")
root = tk.Tk()
root.title("Tkinter 示例")
btn_hello = tk.Button(root, text="点击我", command=say_hello)
btn_hello.pack()
root.mainloop()
```
在这个示例中,我们首先导入了`tkinter`模块,然后创建了一个简单的窗口(通过`Tk()`)并给这个窗口设置了标题。接着,我们创建了一个按钮(`Button`),并指定了按钮上显示的文本,以及点击按钮时要调用的函数(`command=say_hello`)。使用`pack()`方法将按钮添加到窗口中,并通过`mainloop()`启动事件循环。
在构建图形化界面时,通常需要定义窗口、小部件、布局及事件处理等元素,Python通过其简洁的语法能够使得这一过程变得相对简单。
#### 2.1.2 Python图形化工具的常用命令
在Python的Tkinter库中,我们常用的一些命令包括用于创建小部件的命令,如`Button`、`Label`、`Entry`等。除此之外,还有一系列用于管理小部件布局的几何管理器命令,例如`pack()`、`grid()`和`place()`。
- `pack()`:通过将小部件打包进容器来进行布局,它会自动处理对齐和边距。
- `grid()`:使用网格来组织小部件,提供了更大的灵活性来控制小部件的位置。
- `place()`:以绝对坐标的方式定位小部件,允许开发者指定小部件的确切位置。
此外,每个小部件都有其特定的属性设置方法,例如设置背景色、字体等,这些都是通过命令形式的函数来实现的。
### 2.2 Python图形化工具的数据处理
#### 2.2.1 数据的读取和解析
在Python图形化工具中,数据的读取和解析是前期准备工作的重要部分。通常,数据可以从文件、网络或数据库等多种来源获取。一旦获取数据,下一步就是解析这些数据以供可视化使用。
以下是一个从CSV文件中读取数据并解析的示例:
```python
import csv
# 打开CSV文件
with open('data.csv', mode='r') as ***
* 创建CSV阅读器
reader = csv.reader(file)
# 跳过标题行
next(reader)
# 读取每一行数据
for row in reader:
# 解析数据
print(row)
```
在这个例子中,我们使用Python的内置`csv`模块来打开和读取一个CSV文件。首先,我们创建了一个CSV阅读器,然后跳过标题行,最后循环读取每一行数据,并将其打印出来。
#### 2.2.2 数据的转换和处理
在实际应用中,通常需要对原始数据进行一些转换和处理才能用于可视化。数据处理包括数据清洗、类型转换、数据汇总和聚合等多种操作。
假设我们需要对上述CSV文件中读取的数据进行统计分析,可以使用Python的`collections`模块中的`Counter`类来计算数据的频率:
```python
from collections import Counter
# 假设CSV文件的某列包含需要计数的数据
with open('data.csv', mode='r') as ***
***
*** 跳过标题行
data = [row[1] for row in reader] # 选择第二列数据
counter = Counter(data)
print(counter)
```
在这段代码中,我们从CSV文件的第二列提取数据,并使用`Counter`对数据中的元素出现频率进行计数,最后打印出每个元素的频率。
### 2.3 Python图形化工具的网络数据可视化
#### 2.3.1 网络数据可视化的原理
网络数据可视化是对网络结构和网络流量等抽象数据的图形表示。可视化可以揭示模式、趋势和异常等特征,帮助人们理解和分析数据。网络数据可视化通常基于图论的原理,图由节点和边组成,节点代表实体,边代表实体间的某种关系。
在Python中,我们可以利用图论库如`networkx`来创建和操作图对象,然后使用图形库如`matplotlib`来进行可视化。
下面是一个生成一个简单的网络图并可视化的例子:
```python
import networkx as nx
import matplotlib.pyplot as plt
# 创建一个图对象
G = nx.Graph()
# 添加节点
G.add_node(1)
G.add_node(2)
G.add_node(3)
# 添加边
G.add_edge(1, 2)
G.add_edge(1, 3)
# 绘制图形
nx.draw(G, with_labels=True)
plt.show()
```
这个例子创建了一个简单的无向图,并用`networkx`库来定义图的结构,然后用`matplotlib`库来绘制图。
#### 2.3.2 网络数据可视化的实现方法
实现网络数据可视化,除了使用`networkx`和`matplotlib`之外,还可以使用专门的可视化库如`Graphviz`、`Gephi`或`Cytoscape`等,它们提供了更多的定制化选项。
下面是一个使用`Graphviz`进行网络数据可视化的例子:
首先安装Graphviz库:
```bash
pip install graphviz
```
然后编写代码创建一个网络图并保存为图像文件:
```python
from graphviz import Digraph
dot = Digraph(comment='The Test Table')
dot.node('A', 'Alice')
dot.node('B', 'Bob')
dot.node('C', 'Charlie')
dot.edges(['AB', 'AC'])
dot.render('test-output/test-table.gv', view=True)
```
上述代码创建了一个有向图,并将图渲染为一个图像文件。`render`方法会调用Graphviz的可执行文件,将图输出为PNG格式的图像。
### 2.3.3 网络数据可视化的实现方法
网络数据可视化涉及数据的采集、处理、分析,最终转换成可视化的图形。这里,我们将探讨使用Python进行网络数据可视化的几种主要方法。
1. **使用`networkx`和`matplotlib`**
`networkx`是一个功能强大的图论和网络分析工具,它提供了创建、操作以及绘制网络图的接口。与`matplotlib`结合,`networkx`可以将复杂的数据关系以图形的形式直观展现出来。
```python
import networkx as nx
import matplotlib.pyplot as plt
G = nx.Graph() # 创建一个空的无向图
G.add_edge(1, 2)
G.add_edge(1, 3)
G.add_edge(2, 4)
G.add_edge(3, 4)
pos = nx.spring_layout(G) # 获得图形布局
nx.draw(G, pos, with_labels=True, node_color='skyblue', edge_color='black') # 绘制图形
plt.show() # 显示图形
```
2. **使用`Graphviz`**
`Graphviz` 是一个图形可视化软
0
0
相关推荐
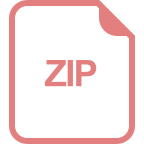
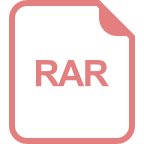





