Python中的树与森林:拓扑数据结构的实现与优化
发布时间: 2024-09-11 16:17:50 阅读量: 139 订阅数: 80 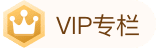
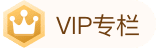
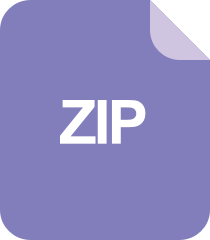
杭电的2011-2015数据结构考研题解.zip
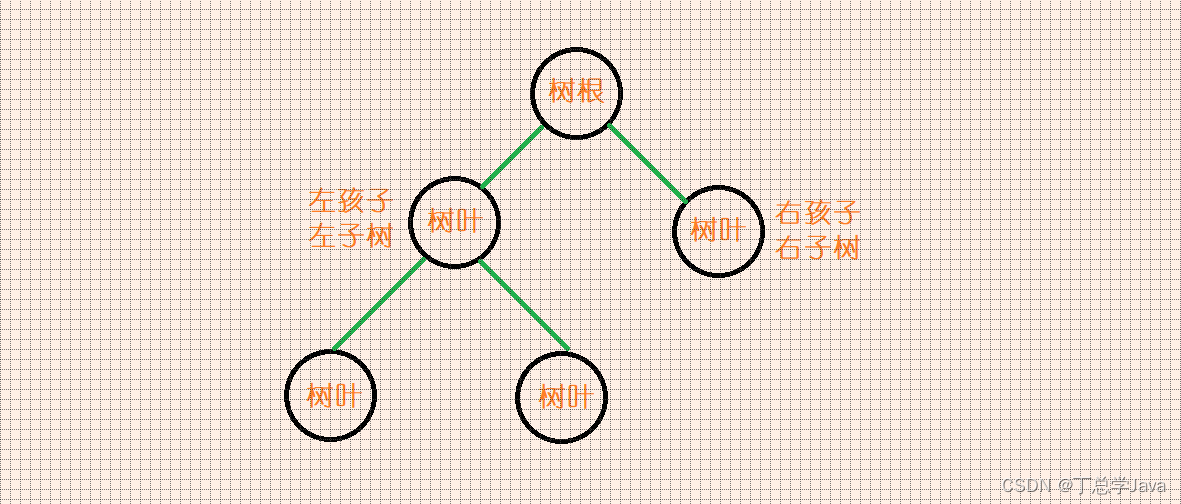
# 1. 树与森林数据结构概述
数据结构是计算机存储、组织数据的方式,它决定了数据处理的效率和复杂度。在数据结构的世界里,树与森林是两个密切相关且极其重要的概念。
## 1.1 树与森林的定义
在计算机科学中,树是一种抽象数据类型(ADT),或者称为一种具有层次关系的数据结构。它模拟了自然界中的树结构,具有一个根节点,以及零个或多个子节点。树结构用于表示具有层次关系的数据,如文件系统的目录结构、组织架构图等。
森林则可被看作是由多棵树组成的集合,在某些情况下,森林也可以视为没有根节点的树。在图论中,森林是一种特殊的图,它是一个无环连通图。这意味着在森林中任意两个节点之间只有一条路径相连,不存在环。
## 1.2 树与森林的应用场景
由于树与森林结构的层次性和易扩展性,它们在多种应用场景中扮演着重要角色。例如,在关系数据库中,树形结构常被用于表示数据之间的层级关系,如公司组织架构或产品分类。在编程语言的编译器中,语法分析树是理解程序结构的关键。同时,森林也是许多复杂算法,如哈夫曼编码、LZW压缩算法等的基石。
下一章将深入探讨树的理论基础和遍历算法,我们将从树的基本概念开始,逐步深入理解树的数据结构特性,并掌握其遍历技术。
# 2. 树的理论基础和遍历算法
### 2.1 树的基本概念和性质
#### 2.1.1 树的定义与分类
在计算机科学领域,树是一种重要的非线性数据结构,它模拟了具有层次关系的结构。树由节点组成,每个节点都可能有一个或多个子节点,而只有一个节点是根节点,它没有父节点。
树可以按照不同的标准分类。例如,根据节点的子节点数量,我们可以将其分为:无节点树(空树)、单节点树、二叉树(每个节点最多有两个子节点)、k叉树(每个节点最多有k个子节点)等。树的这些属性定义了它们的形状和行为,影响了树的操作效率和使用场景。
```mermaid
graph TD
A[根节点] --> B[子节点1]
A --> C[子节点2]
A --> D[子节点3]
B --> E[孙节点1]
B --> F[孙节点2]
C --> G[孙节点3]
D --> H[孙节点4]
```
在这个mermaid流程图中,我们可以看到一个简单的树结构的可视化表示,其中根节点A拥有三个子节点B、C和D,而子节点B又拥有自己的两个子节点E和F。
#### 2.1.2 树的数学表示和属性
树在数学上可以通过递归关系来表示。一个树可以看做是n个子树的集合,其中每个子树又是一棵树。树的数学表示和属性包括节点数、边数、树的高度等。
- **节点数**(N):树中节点的数量。
- **边数**(E):树中边的数量总是节点数减一,即E = N - 1。
- **树的高度**(H):从根节点到最远叶子节点的最长路径上的边数。
这些属性是分析树操作复杂度和进行算法设计时的基础。
### 2.2 二叉树及其特殊形态
#### 2.2.1 完全二叉树和满二叉树
完全二叉树是一种特殊的二叉树,其中每个层的节点都是满的,除了可能的最后一层。在最后一层,节点从左到右填充。满二叉树是指所有层都是满的二叉树。
完全二叉树和满二叉树在很多算法中非常重要,例如堆排序和优先队列的实现,它们在物理存储上通常可以使用数组来实现,这样可以快速访问父节点和子节点。例如,给定一个节点的索引i,其左子节点的索引是2*i + 1,右子节点的索引是2*i + 2。
```python
def left_child(index):
return 2 * index + 1
def right_child(index):
return 2 * index + 2
def parent(index):
if index > 0:
return (index - 1) // 2
else:
return None
```
这些函数允许我们在不使用指针的情况下,在数组中快速定位父节点和子节点。
#### 2.2.2 平衡二叉树和B树系列
平衡二叉树(如AVL树)是一种自平衡的二叉搜索树,其中任何节点的两个子树的高度差都不超过1。平衡二叉树确保了搜索操作的最坏情况下的时间复杂度保持在O(log n)。
B树是一种平衡的多路查找树,它能够保持数据有序,并允许搜索、顺序访问、插入和删除在对数时间内完成。B树特别适合读写相对较大的数据块的系统,如磁盘存储。
### 2.3 树的遍历技术
#### 2.3.1 前序、中序、后序遍历
树的遍历是指访问树中每个节点一次且仅一次的过程。遍历可以分为前序遍历、中序遍历和后序遍历。
- **前序遍历**:首先访问根节点,然后递归地进行前序遍历左子树,接着递归地进行前序遍历右子树。
- **中序遍历**:首先递归地进行中序遍历左子树,然后访问根节点,最后递归地进行中序遍历右子树。
- **后序遍历**:首先递归地进行后序遍历左子树,然后递归地进行后序遍历右子树,最后访问根节点。
这些遍历方法可以用来输出树的所有节点,或者用于计算表达式树的值等。
```python
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def preorder_traversal(root):
if root:
print(root.value, end=' ')
preorder_traversal(root.left)
preorder_traversal(root.right)
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.value, end=' ')
inorder_traversal(root.right)
def postorder_traversal(root):
if root:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.value, end=' ')
# 示例树的构造
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
# 执行遍历
print("Preorder: ", end='')
preorder_traversal(root)
print("\nInorder: ", end='')
inorder_traversal(root)
print("\nPostorder: ", end='')
postorder_traversal(root)
```
在上面的代码中,我们定义了一个简单的二叉树,并展示了如何实现和执行前序、中序和后序遍历。
#### 2.3.2 层次遍历与广度优先搜索(BFS)
层次遍历是指按照树的层次从上到下、从左到右访问树的节点。广度优先搜索(BFS)使用的就是层次遍历的方法,它适用于查找最短路径或者在无权图中查找两个节点的最短路径。
在实现层次遍历时,可以使用队列来按顺序处理每一层的节点。每处理完一层,就可以将其子节点加入队列中,这样就可以保证按照层次顺序来访问节点。
```python
from collections import deque
def level_order_traversal(root):
if not root:
return
queue = deque([root])
while queue:
node = queue.popleft()
print(node.value, end=' ')
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
# 使用上面构建的示例树执行层次遍历
print("Level Order: ", end='')
level_order_traversal(root)
```
层次遍历的代码逻辑清楚地展示了队列如何用来实现按照层次顺序访问树的所有节点。通过逐层处理,我们能够有
0
0
相关推荐







