java swing 界面显示 选择文件框选并且选择的文件显示,下面 ip地址输入框 端口号输入框,端口测试按钮 ,实现文件传输
时间: 2024-05-06 16:20:02 浏览: 101
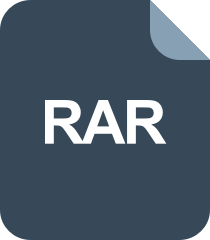
java实现文件传输

以下是一个简单的Java Swing界面,包含选择文件框、IP地址输入框、端口号输入框和端口测试按钮。选择文件后,文件路径会显示在界面上。点击端口测试按钮会尝试连接输入的IP地址和端口号,如果连接成功,会弹出对话框显示“连接成功”,否则会显示“连接失败”。
```
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.EmptyBorder;
public class FileTransferUI extends JFrame {
private JPanel contentPane;
private JTextField ipTextField;
private JTextField portTextField;
private JTextField fileTextField;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FileTransferUI frame = new FileTransferUI();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public FileTransferUI() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel lblNewLabel = new JLabel("IP地址:");
lblNewLabel.setBounds(10, 10, 54, 15);
contentPane.add(lblNewLabel);
ipTextField = new JTextField();
ipTextField.setBounds(74, 7, 100, 21);
contentPane.add(ipTextField);
ipTextField.setColumns(10);
JLabel lblNewLabel_1 = new JLabel("端口号:");
lblNewLabel_1.setBounds(184, 10, 54, 15);
contentPane.add(lblNewLabel_1);
portTextField = new JTextField();
portTextField.setBounds(248, 7, 66, 21);
contentPane.add(portTextField);
portTextField.setColumns(10);
JButton testBtn = new JButton("端口测试");
testBtn.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String ip = ipTextField.getText().trim();
String portStr = portTextField.getText().trim();
int port = Integer.parseInt(portStr);
boolean success = FileTransferUtil.testConnection(ip, port);
if (success) {
JOptionPane.showMessageDialog(FileTransferUI.this, "连接成功");
} else {
JOptionPane.showMessageDialog(FileTransferUI.this, "连接失败");
}
}
});
testBtn.setBounds(324, 6, 93, 23);
contentPane.add(testBtn);
JButton fileBtn = new JButton("选择文件");
fileBtn.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
JFileChooser chooser = new JFileChooser();
int result = chooser.showOpenDialog(FileTransferUI.this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = chooser.getSelectedFile();
fileTextField.setText(file.getAbsolutePath());
}
}
});
fileBtn.setBounds(10, 50, 93, 23);
contentPane.add(fileBtn);
fileTextField = new JTextField();
fileTextField.setEditable(false);
fileTextField.setBounds(113, 50, 304, 21);
contentPane.add(fileTextField);
fileTextField.setColumns(10);
}
}
```
其中,`FileTransferUtil`是一个工具类,用于测试连接,具体实现可以根据需要自行编写。`JOptionPane`是Java Swing提供的对话框组件,用于显示连接成功或失败的信息。
阅读全文
相关推荐
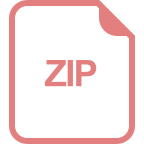
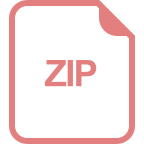
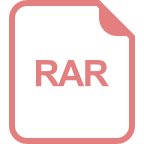
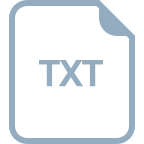
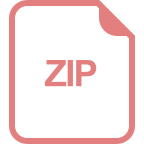
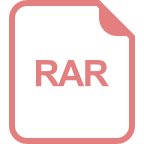
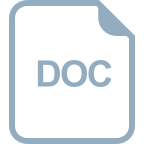
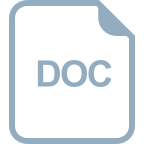


