用python实现对图像基于阈值的区域生长代码
时间: 2023-12-10 11:40:11 浏览: 40
以下是基于阈值的区域生长的Python代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.png', 0)
# 定义阈值和种子点
threshold = 100
seed_point = (50, 50)
# 区域生长
region = set()
region.add(seed_point)
while len(region) > 0:
# 取出一个点
current_point = region.pop()
# 获取当前点的相邻像素
neighbors = [(current_point[0]+1, current_point[1]),
(current_point[0]-1, current_point[1]),
(current_point[0], current_point[1]+1),
(current_point[0], current_point[1]-1)]
# 遍历相邻像素
for neighbor in neighbors:
# 判断相邻像素是否在图像范围内
if neighbor[0] >= img.shape[0] or neighbor[1] >= img.shape[1]:
continue
# 判断相邻像素是否已经被访问过
if neighbor in region:
continue
# 判断相邻像素与种子点之间的差值是否小于阈值
if abs(int(img[current_point]) - int(img[neighbor])) < threshold:
region.add(neighbor)
img[neighbor] = 255
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个代码中,我们首先读取一张灰度图像,然后定义了阈值和种子点。接着,我们使用集合来存储当前区域的像素点,并将种子点添加到集合中。然后进入循环,每次从集合中取出一个点,遍历它的相邻像素,判断是否符合区域生长的条件,如果符合,则将相邻像素加入到集合中,并将其像素值设为255。最后,显示结果图像。
请注意,这个代码只是一个基本的实现,可能无法处理复杂的图像。在实际应用中,您可能需要对代码进行优化或使用其他算法来进行区域生长。
相关推荐












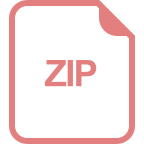
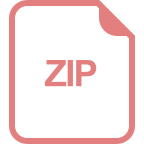
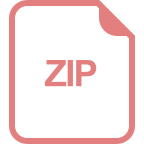