Unity 循环滑动列表
时间: 2024-04-30 18:20:58 浏览: 160
在 Unity 中实现循环滑动列表可以使用 ScrollRect 组件和 ContentSizeFitter 组件。以下是一个基本的步骤:
1. 创建一个空白 GameObject,并向其中添加 ScrollRect 组件。
2. 在 ScrollRect 组件中设置 Content 属性为一个包含所有列表项的 GameObject。
3. 向 Content 中添加一个 VerticalLayoutGroup 组件,用于设置列表项的垂直布局。
4. 向每个列表项 GameObject 中添加一个 LayoutElement 组件,用于设置列表项的高度。
5. 向 ScrollRect 中添加一个 ContentSizeFitter 组件,并将 Vertical Fit 设置为 Preferred Size。
6. 编写一个脚本,用于控制滑动列表的循环滚动。该脚本应该包含以下步骤:
- 在 Start() 方法中获取列表项的总数和高度。
- 在 Update() 方法中检查 ScrollRect 是否已经滚动到列表的顶部或底部,并相应地更新 Content 的位置以实现循环滚动。
下面是一个简单的示例代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class LoopScroll : MonoBehaviour
{
public float itemHeight; // 列表项的高度
public int itemCount; // 列表项的总数
private RectTransform content;
private ScrollRect scrollRect;
private float contentHeight;
private void Start()
{
content = GetComponent<ScrollRect>().content;
scrollRect = GetComponent<ScrollRect>();
contentHeight = itemHeight * itemCount;
content.sizeDelta = new Vector2(content.sizeDelta.x, contentHeight);
}
private void Update()
{
if (scrollRect.verticalNormalizedPosition <= 0f)
{
content.anchoredPosition = new Vector2(content.anchoredPosition.x, content.anchoredPosition.y + contentHeight);
scrollRect.verticalNormalizedPosition = 1f;
}
else if (scrollRect.verticalNormalizedPosition >= 1f)
{
content.anchoredPosition = new Vector2(content.anchoredPosition.x, content.anchoredPosition.y - contentHeight);
scrollRect.verticalNormalizedPosition = 0f;
}
}
}
```
在这个示例中,我们在 Start() 方法中计算了 Content 的总高度,并在 Update() 方法中检查 ScrollRect 是否已经滚动到列表的顶部或底部。如果是,我们就相应地更新 Content 的位置以实现循环滚动。注意,我们还将 ScrollRect 的 verticalNormalizedPosition 设置为 0f 或 1f,以保持滚动位置不变。
阅读全文
相关推荐
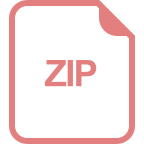
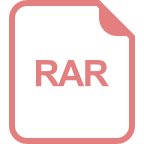
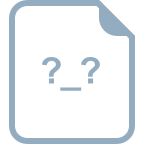
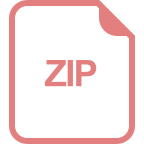
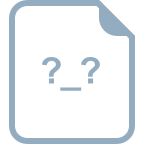




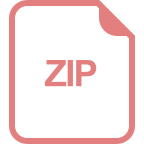
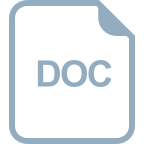
