线程池计算素数:编写一个程序primer_thr3.c,程序运行开始时,创建10个子线程和一个包含20个元素的队列,主线程不断地依次将30000000到30000200之间这200个数放入队列,如果队列满,则主线程等待。
时间: 2024-02-12 19:05:34 浏览: 68
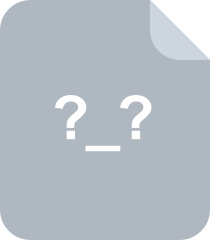
编写程序:计算100-10000之间有多少个素数,并输出所有素数。
好的,这是一个比较复杂的问题,但我会尽力回答。首先,需要注意的是,这是一个多线程的程序,需要使用线程池来管理线程。线程池可以通过预先创建一定数量的线程来避免频繁地创建和销毁线程,从而提高程序的效率。
以下是实现该程序的基本步骤:
1. 定义一个任务结构体,包含一个待计算的数值和一个标志位,表示该数值是否为素数。
```c
typedef struct {
int num;
int is_prime;
} task_t;
```
2. 定义一个线程池,包含一个任务队列和一定数量的线程。
```c
typedef struct {
task_t *tasks;
int capacity;
int front;
int rear;
pthread_mutex_t lock;
pthread_cond_t not_empty;
pthread_cond_t not_full;
pthread_t *threads;
int thread_count;
int stop;
} thread_pool_t;
```
3. 实现线程池的初始化函数init_thread_pool,其中包括创建任务队列、初始化线程和启动线程等步骤。
```c
void init_thread_pool(thread_pool_t *pool, int capacity, int thread_count) {
pool->tasks = malloc(capacity * sizeof(task_t));
pool->capacity = capacity;
pool->front = 0;
pool->rear = 0;
pthread_mutex_init(&pool->lock, NULL);
pthread_cond_init(&pool->not_empty, NULL);
pthread_cond_init(&pool->not_full, NULL);
pool->threads = malloc(thread_count * sizeof(pthread_t));
pool->thread_count = thread_count;
pool->stop = 0;
for (int i = 0; i < thread_count; i++) {
pthread_create(&pool->threads[i], NULL, thread_func, pool);
}
}
```
4. 实现线程函数thread_func,其中包括从任务队列中取出任务、计算素数和回传结果等步骤。
```c
void *thread_func(void *arg) {
thread_pool_t *pool = (thread_pool_t *) arg;
while (1) {
pthread_mutex_lock(&pool->lock);
while (pool->front == pool->rear && !pool->stop) {
pthread_cond_wait(&pool->not_empty, &pool->lock);
}
if (pool->stop) {
pthread_mutex_unlock(&pool->lock);
pthread_exit(NULL);
}
task_t task = pool->tasks[pool->front];
pool->front = (pool->front + 1) % pool->capacity;
pthread_cond_signal(&pool->not_full);
pthread_mutex_unlock(&pool->lock);
task.is_prime = is_prime(task.num);
// 回传计算结果
pthread_mutex_lock(&result_lock);
results[task.num - start] = task.is_prime;
pthread_mutex_unlock(&result_lock);
}
return NULL;
}
```
5. 实现主函数main,其中包括创建线程池、循环向任务队列中添加任务、等待所有任务完成和销毁线程池等步骤。
```c
int main() {
// 创建线程池
thread_pool_t pool;
init_thread_pool(&pool, QUEUE_SIZE, THREAD_COUNT);
// 循环向任务队列中添加任务
for (int i = start; i <= end; i++) {
task_t task = {i, 0};
pthread_mutex_lock(&pool.lock);
while ((pool.rear + 1) % pool.capacity == pool.front) {
pthread_cond_wait(&pool.not_full, &pool.lock);
}
pool.tasks[pool.rear] = task;
pool.rear = (pool.rear + 1) % pool.capacity;
pthread_cond_signal(&pool.not_empty);
pthread_mutex_unlock(&pool.lock);
}
// 等待所有任务完成
while (1) {
pthread_mutex_lock(&pool.lock);
if (pool.front == pool.rear) {
pthread_mutex_unlock(&pool.lock);
break;
}
pthread_mutex_unlock(&pool.lock);
}
// 销毁线程池
pool.stop = 1;
pthread_cond_broadcast(&pool.not_empty);
for (int i = 0; i < pool.thread_count; i++) {
pthread_join(pool.threads[i], NULL);
}
pthread_mutex_destroy(&pool.lock);
pthread_cond_destroy(&pool.not_empty);
pthread_cond_destroy(&pool.not_full);
free(pool.tasks);
free(pool.threads);
return 0;
}
```
以上是一个基本的实现,当然还有很多细节需要处理,比如如何确定素数的算法、如何保证线程安全等问题。但是希望这个基本的框架可以帮助你理解如何使用线程池编写多线程程序。
阅读全文
相关推荐



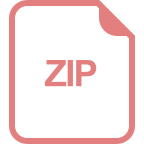
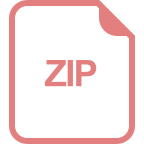