单链表基础应用(2)--插入一个结点c语言
时间: 2023-06-03 09:04:27 浏览: 96
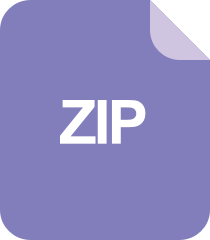
C语言实现单链表(带头结点)的基本操作

好的,关于单链表的基础应用,我可以帮你解答。在单链表中插入一个结点,首先要有指向头节点的指针,以便能够操作链表。假设链表中已经有了若干个节点,现在我们要在中间插入一个新节点。具体步骤如下:
1. 创建一个新节点,将待插入的数据存储在这个节点中;
2. 找到待插入节点的前一个节点,将其指针域指向新节点,同时将新节点的指针域指向待插入节点;
3. 若待插入节点是链表尾部,则直接将新节点插入到链表尾部即可。
这是单链表中插入节点的基本过程,具体实现可以根据代码要求进行调整。希望能够对你有所帮助。
阅读全文
相关推荐
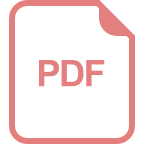


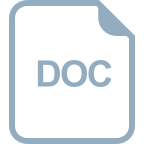












