tic [Model, Path] = myDstarLite(Model); Sol = Path; Sol.pTime = toc; Sol.cost = costL(Sol.coords); Sol.smoothness = smoothness(Sol.coords); % [Sol.cost, Sol.solChar]= costLinear(Model, Sol.coords);
时间: 2024-03-28 20:36:22 浏览: 43
这段代码看起来像是在使用D* Lite算法来解决路径规划问题。具体来说,该算法会利用一个地图模型(Model)和起点与终点的坐标信息来找到一条最优路径(Path)。其中,toc函数用于计算程序运行的时间,costL和smoothness函数则用于计算路径的代价和平滑度。最后,代码会返回一个包含路径信息的结构体Sol,其中包括路径的坐标、代价、平滑度以及计算时间等信息。
相关问题
请以解决多AGV路径规划为目的改进下面的代码% D*Lite: Path Planning Algorithm - MATLAB % Main code for running the algorithm. % Morteza Haghbeigi, m.haghbeigi@gmail.com % Initialization clc clear close %% settings Model.expandMethod = 'random'; % random or heading 随机走还是先向上走 Model.distType = 'manhattan'; % euclidean or manhattan; Model.adjType = '4adj'; % 4adj or 8adj %% Create Map and Model by User 按用户创建地图模型 % createModelBaseEmpty createModelBase Model=createModelBase(Model); Model=createModelDstarLite(Model); %% # optimal path by Astar A星算法下的最优路径 tic [Model, Path] = myDstarLite(Model); Sol = Path; Sol.pTime = toc; Sol.cost = costL(Sol.coords); Sol.smoothness = smoothness(Sol.coords); % [Sol.cost, Sol.solChar]= costLinear(Model, Sol.coords); %% display data and plot solution disp(['process time for path= ' num2str(Sol.pTime)]) disp(Sol) plotModel(Model) plotSolution(Sol.coords, []) % plotAnimation2(Sol.coords) %% clear temporal data clear adj_type dist_type
首先,需要明确多AGV路径规划的具体要求和限制,包括AGV数量、起点和终点位置、地图信息等。然后根据这些要求和限制,将当前代码进行改进,使其可以解决多AGV路径规划问题。
具体改进建议如下:
1. 修改地图模型:在当前代码的基础上,需要增加AGV的位置和状态信息,包括每个AGV的当前位置、目标位置和运动状态等。可以采用二维数组或者结构体等方式进行存储。
2. 修改路径搜索算法:由于需要考虑多个AGV的运动,因此需要修改路径搜索算法,使其可以同时搜索多个AGV的路径,并且保证路径不重叠、不冲突。可以采用扩展D*算法等高效的路径搜索算法进行改进。
3. 增加路径规划策略:在多AGV路径规划中,需要考虑到不同AGV之间的协同和调度,以最小化路径长度、时间等指标。可以采用基于协同优化的路径规划策略,如集中式、分布式、协作式等方式进行改进。
4. 增强可视化功能:为了方便用户进行路径规划和调试,需要增强可视化功能,如增加AGV的图标、路径的颜色、动画展示等,使用户可以直观地了解路径规划的结果和过程。
综上所述,针对多AGV路径规划问题,需要对当前代码进行全面的改进和优化,以提高路径规划的效率和准确性。
这段代码优化巡行时间:clc;clear; tic %遍历循环读取所有tiff文件 file_path = 'D:\JIANGXiaoYu\5_20230709_24dpf\';% 图像文件夹路径 img_path_list = dir(strcat(file_path,'*.tiff'));%获取该文件夹中所有TIFF格式的图像 img_num = length(img_path_list);%获取图像总数量 Output_path='D:\JIANGXiaoYu\5_20230709_24dpf_tif\';%文件夹的路径 for jj = 1:img_num image_name = img_path_list(jj).name; % 图像名 image=imread(strcat(file_path,img_path_list(jj).name)); Info=imfinfo(image_name); Slice=size(Info,1); %%获取图片z向帧数 Width=Info.Width; Height=Info.Height; Image=zeros(Height,Width,Slice); for i=1:Slice Image(:,:,i)=imread(strcat(file_path,img_path_list(jj).name),i); %%一层一层的读入图像 J=uint8(Image(:,:,i)); %%一层一层写出图像 imwrite(J,[Output_path,num2str(209*(jj-1)+i,'%04d'),'.tif']);%2G209/4G419 end fprintf(' %d %s\n',jj,strcat(file_path,image_name));% 显示正在处理的图像名 end toc disp(['运行时间: ',num2str(toc)]); %遍历循环读取所有tiff文件
这段代码的主要功能是遍历指定文件夹中的所有TIFF图像,并将每个图像的每一层保存为单独的文件。为了优化巡行时间,可以考虑以下几点:
1. 减少磁盘IO操作:当前代码在每次循环中都会读取和写入图像,这会带来较大的IO开销。可以尝试将所有图像先读取到内存中,然后再进行写入操作,这样可以减少IO次数。
2. 并行处理:使用并行计算可以提高代码的运行效率。可以考虑使用parfor循环来并行处理每个图像的每一层。
下面是对代码进行优化的示例:
clc;
clear;
tic
file_path = 'D:\JIANGXiaoYu\5_20230709_24dpf\';
img_path_list = dir(fullfile(file_path, '*.tiff'));
img_num = length(img_path_list);
output_path = 'D:\JIANGXiaoYu\5_20230709_24dpf_tif\';
parfor jj = 1:img_num
image_name = img_path_list(jj).name;
image = imread(fullfile(file_path, image_name));
Info = imfinfo(fullfile(file_path, image_name));
slice = size(Info, 1);
width = Info.Width;
height = Info.Height;
temp_images = zeros(height, width, slice);
for i = 1:slice
temp_images(:, :, i) = imread(fullfile(file_path, image_name), i);
end
for i = 1:slice
J = uint8(temp_images(:, :, i));
imwrite(J, fullfile(output_path, [num2str(209 * (jj - 1) + i, '%04d'), '.tif']));
end
fprintf(' %d %s\n', jj, fullfile(file_path, image_name));
end
toc
disp(['运行时间: ', num2str(toc)]);
阅读全文
相关推荐
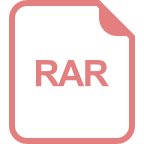
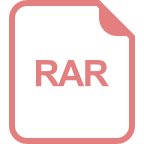
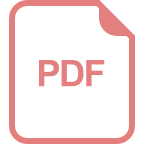
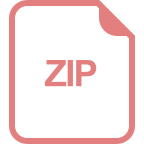
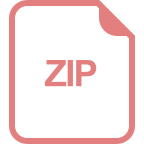
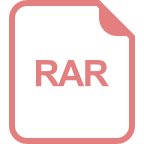
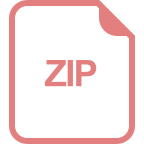
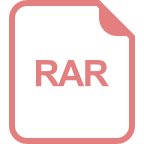
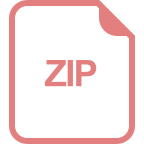
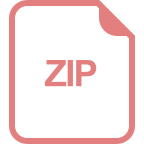
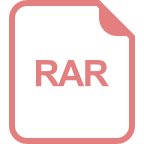
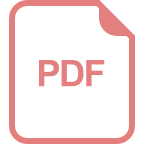
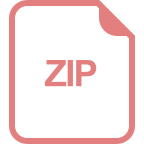
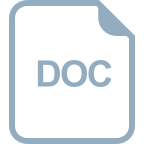
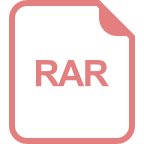
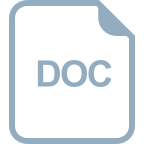
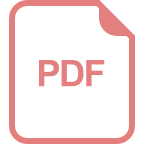