#include <iostream> using namespace std; void extractNum(char * str); int main() { char s[1024]; cin.getline(s,1024); // 输入一行字符 extractNum(s); // 调用extractNum函数,选出数字 cout<<s<<endl; // 输出选出的数字 return 0; } // 函数extractNum:选出str指向的字符串中的数字,并写回str // 参数:str-指向字符串 void extractNum(char * str) { // 请在此添加代码,实现函数extractNum /********** Begin *********/ char *p=str; char *q=str; bool m=false; while(*q!='\0') { if(!m && *q=='-') { *p='-'; p++; m=true; } else if(*q<='9' && *q>='0') { *p=*q; p++; m=true; } q++; } *p='\0'; /********** End **********/ } /********** End **********/
时间: 2023-07-18 20:46:36 浏览: 29
这段代码是一个提取字符串中数字的程序,主函数中通过输入一行字符,调用函数extractNum来选出数字并输出。函数extractNum的实现思路是利用两个指针p和q,p指向选出的数字存放的位置,q遍历字符串中的每个字符,当q指向数字字符时将其存入p指向的位置中。同时,当字符为负号时将其存入p指向的位置中,并将m标记为true,表示已经存入了符号。最后将p指向的位置置为'\0',表示字符串结束。
相关问题
#include <iostream> using namespace std; void extractNum(char * str); int main() { char s[1024]; cin.getline(s,1024); // 输入一行字符 extractNum(s); // 调用extractNum函数,选出数字 cout<<s<<endl; // 输出选出的数字 return 0; } // 函数extractNum:选出str指向的字符串中的数字,并写回str // 参数:str-指向字符串 void extractNum(char * str) { // 请在此添加代码,实现函数extractNum ....... }
以下是一个实现 extractNum 函数的示例代码:
```
#include <iostream>
using namespace std;
void extractNum(char * str);
int main()
{
char s[1024];
cin.getline(s, 1024); // 输入一行字符
extractNum(s); // 调用extractNum函数,选出数字
cout << s << endl; // 输出选出的数字
return 0;
}
// 函数extractNum:选出str指向的字符串中的数字,并写回str
// 参数:str-指向字符串
void extractNum(char * str)
{
int i = 0, j = 0;
char temp[1024]; // 用于存放选出的数字
while (str[i] != '\0')
{
if (isdigit(str[i]))
{
temp[j] = str[i];
j++;
}
i++;
}
temp[j] = '\0'; // 手动添加字符串结尾符
strcpy(str, temp); // 将选出的数字复制回原字符串
}
```
该函数使用了一个 while 循环来遍历字符串中的每个字符,如果该字符是数字,则将其存入一个临时数组中。最后,手动添加字符串结尾符 '\0' 后,将选出的数字复制回原字符串中。
#include <iostream> #include <cstring> using namespace std; void extractNum(char * str); int main(){ char s[1024]; cin.getline(s,1024); // 输入一行字符 extractNum(s); // 调用extractNum函数,选出数字 cout<<s<<endl; // 输出选出的数字 return 0; } // 函数extractNum:选出str指向的字符串中的数字,并写回str // 参数:str-指向字符串 void extractNum(char * str){ // 请在此添加代码,实现函数extractNum /********** Begin *********/ char s1[1024]; char *a=s1; while(*str!='\0'){ if(*str<='9'&&*str>='0'){ *a=*str; a++; str++; } } *a='\0'; str=&s1; /********** End **********/ }这段代码运行超时,请指出其错误并改正
这段代码的错误在于函数extractNum中的指针str被赋值为s1的地址,而s1是一个局部变量,函数结束后s1的内存空间会被释放,指针str指向的地址也不再有效。因此,需要将选出的数字写回原字符串,可以直接在原字符串上进行操作,不需要额外定义一个字符数组。
下面是修改后的代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void extractNum(char * str);
int main(){
char s[1024];
cin.getline(s,1024); // 输入一行字符
extractNum(s); // 调用extractNum函数,选出数字
cout<<s<<endl; // 输出选出的数字
return 0;
}
// 函数extractNum:选出str指向的字符串中的数字,并写回str
// 参数:str-指向字符串
void extractNum(char * str){
// 请在此添加代码,实现函数extractNum
/********** Begin *********/
char *p = str; // 定义指针p指向原字符串
while(*str != '\0'){
if(*str >= '0' && *str <= '9'){
*p = *str; // 将数字写回原字符串
p++; // 指针p向后移动
}
str++; // 指针str向后移动
}
*p = '\0'; // 将原字符串中非数字部分的字符设为'\0'
/********** End **********/
}
```
相关推荐
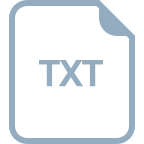
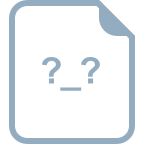
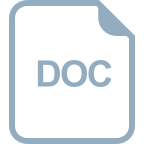












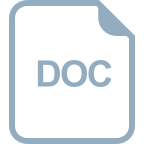
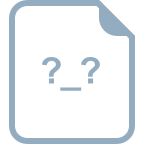