针对LV8728MR驱动步进电机的加速启动和减速停止的C语言程序
时间: 2023-12-27 09:03:45 浏览: 228
以下是一个简单的 C 语言程序,可用于控制 LV8728MR 驱动步进电机的加速启动和减速停止。
首先需要定义一些常量和变量:
```c
#define ACCEL_RATE 100 // 加速率
#define DECEL_RATE 100 // 减速率
#define MAX_SPEED 1000 // 最大速度
#define MIN_SPEED 200 // 最小速度
#define STEPS_PER_REV 200 // 电机步数
int step_delay; // 步进延迟时间
int current_speed = 0; // 当前速度
int target_speed = 0; // 目标速度
int accel_count = 0; // 加速计数器
int decel_count = 0; // 减速计数器
int step_count = 0; // 步数计数器
int steps_to_cruise = 0; // 加速到最大速度需要的步数
int steps_to_brake = 0; // 减速到停止需要的步数
int cruise_speed = 0; // 巡航速度
int direction = 1; // 方向
```
然后需要初始化 LV8728MR 驱动器:
```c
void setup() {
pinMode(STEP_PIN, OUTPUT);
pinMode(DIR_PIN, OUTPUT);
digitalWrite(DIR_PIN, HIGH);
digitalWrite(STEP_PIN, LOW);
step_delay = 1000;
}
```
接下来是控制电机运动的主要函数:
```c
void moveMotor(int steps) {
// 设置方向
if (steps < 0) {
direction = -1;
digitalWrite(DIR_PIN, LOW);
steps = -steps;
} else {
direction = 1;
digitalWrite(DIR_PIN, HIGH);
}
// 计算加速和减速所需步数
steps_to_cruise = (MAX_SPEED - current_speed) / ACCEL_RATE;
steps_to_brake = current_speed / DECEL_RATE;
// 限制速度在最大和最小值之间
if (target_speed > MAX_SPEED) target_speed = MAX_SPEED;
if (target_speed < MIN_SPEED) target_speed = MIN_SPEED;
// 根据加速度调整速度
if (step_count < steps_to_cruise) {
accel_count++;
current_speed += accel_count * ACCEL_RATE;
} else if (step_count > (steps - steps_to_brake)) {
decel_count++;
current_speed -= decel_count * DECEL_RATE;
} else {
current_speed = target_speed;
}
// 计算步进延迟时间
cruise_speed = (current_speed * 100) / 60;
step_delay = 1000000L / (cruise_speed * STEPS_PER_REV);
// 步进电机
for (int i = 0; i < steps; i++) {
digitalWrite(STEP_PIN, HIGH);
delayMicroseconds(step_delay);
digitalWrite(STEP_PIN, LOW);
delayMicroseconds(step_delay);
step_count++;
}
}
```
在这个函数中,我们首先设置运动的方向,然后计算加速和减速需要的步数,限制速度在最大和最小值之间,并根据加速度调整速度。最后,计算步进延迟时间并控制电机运动。
完整的程序如下:
```c
#define ACCEL_RATE 100 // 加速率
#define DECEL_RATE 100 // 减速率
#define MAX_SPEED 1000 // 最大速度
#define MIN_SPEED 200 // 最小速度
#define STEPS_PER_REV 200 // 电机步数
int step_delay; // 步进延迟时间
int current_speed = 0; // 当前速度
int target_speed = 0; // 目标速度
int accel_count = 0; // 加速计数器
int decel_count = 0; // 减速计数器
int step_count = 0; // 步数计数器
int steps_to_cruise = 0; // 加速到最大速度需要的步数
int steps_to_brake = 0; // 减速到停止需要的步数
int cruise_speed = 0; // 巡航速度
int direction = 1; // 方向
void setup() {
pinMode(STEP_PIN, OUTPUT);
pinMode(DIR_PIN, OUTPUT);
digitalWrite(DIR_PIN, HIGH);
digitalWrite(STEP_PIN, LOW);
step_delay = 1000;
}
void loop() {
// 移动 1000 步
moveMotor(1000);
delay(1000);
// 移动 2000 步
moveMotor(-2000);
delay(1000);
}
void moveMotor(int steps) {
// 设置方向
if (steps < 0) {
direction = -1;
digitalWrite(DIR_PIN, LOW);
steps = -steps;
} else {
direction = 1;
digitalWrite(DIR_PIN, HIGH);
}
// 计算加速和减速所需步数
steps_to_cruise = (MAX_SPEED - current_speed) / ACCEL_RATE;
steps_to_brake = current_speed / DECEL_RATE;
// 限制速度在最大和最小值之间
if (target_speed > MAX_SPEED) target_speed = MAX_SPEED;
if (target_speed < MIN_SPEED) target_speed = MIN_SPEED;
// 根据加速度调整速度
if (step_count < steps_to_cruise) {
accel_count++;
current_speed += accel_count * ACCEL_RATE;
} else if (step_count > (steps - steps_to_brake)) {
decel_count++;
current_speed -= decel_count * DECEL_RATE;
} else {
current_speed = target_speed;
}
// 计算步进延迟时间
cruise_speed = (current_speed * 100) / 60;
step_delay = 1000000L / (cruise_speed * STEPS_PER_REV);
// 步进电机
for (int i = 0; i < steps; i++) {
digitalWrite(STEP_PIN, HIGH);
delayMicroseconds(step_delay);
digitalWrite(STEP_PIN, LOW);
delayMicroseconds(step_delay);
step_count++;
}
}
```
阅读全文
相关推荐





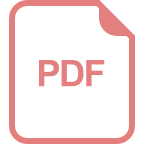

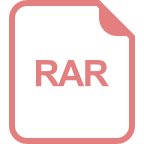





