调用OpenGL三维绘图函数,绘制两个以上的物体(球体茶壶),变换他们的位置。, 2、采用透视变换及正交变换分别对他们进行观察。 3、透视变换中变换观察空间的大小分别对他们进行观察(观测角、近远端截面距离等
时间: 2024-02-05 11:11:54 浏览: 148
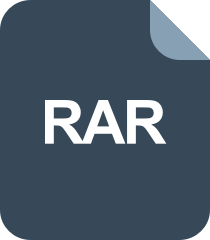
在OpenGL视图中绘制三维物体

以下是使用OpenGL进行三维绘图并变换位置的示例代码:
```c++
#include <GL/glut.h>
// 定义球体和茶壶的位置
GLfloat spherePos[] = {-1.0f, 0.0f, -3.0f};
GLfloat teapotPos[] = {1.0f, 0.0f, -3.0f};
void init() {
// 设置背景色为白色
glClearColor(1.0f, 1.0f, 1.0f, 0.0f);
glEnable(GL_DEPTH_TEST); // 开启深度测试
}
void display() {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// 绘制球体
glPushMatrix(); // 将当前矩阵保存到栈中
glTranslatef(spherePos[0], spherePos[1], spherePos[2]); // 平移球体位置
glutSolidSphere(0.5, 50, 50); // 绘制球体
glPopMatrix(); // 恢复之前保存的矩阵
// 绘制茶壶
glPushMatrix();
glTranslatef(teapotPos[0], teapotPos[1], teapotPos[2]);
glutSolidTeapot(0.5);
glPopMatrix();
glutSwapBuffers();
}
void reshape(int w, int h) {
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
// 正交变换
if (w > h) {
glOrtho(-2.0 * (GLfloat)w / (GLfloat)h, 2.0 * (GLfloat)w / (GLfloat)h, -2.0, 2.0, -10.0, 10.0);
} else {
glOrtho(-2.0, 2.0, -2.0 * (GLfloat)h / (GLfloat)w, 2.0 * (GLfloat)h / (GLfloat)w, -10.0, 10.0);
}
// 透视变换
// gluPerspective(60.0, (GLfloat)w / (GLfloat)h, 1.0, 20.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(400, 400);
glutCreateWindow("3D Objects");
init();
glutDisplayFunc(display);
glutReshapeFunc(reshape);
glutMainLoop();
return 0;
}
```
代码中使用了OpenGL的三维绘图函数glutSolidSphere和glutSolidTeapot分别绘制球体和茶壶,并通过glTranslatef进行平移变换来改变它们的位置。在reshape函数中,可以根据需要选择正交变换或透视变换,通过glOrtho和gluPerspective函数实现。
阅读全文
相关推荐
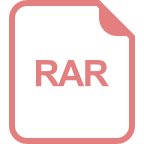




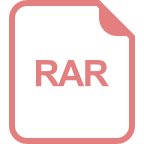
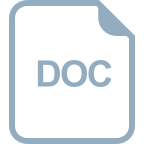
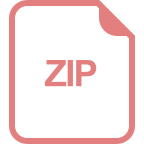
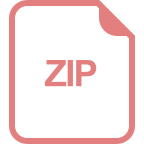
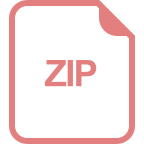
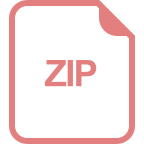
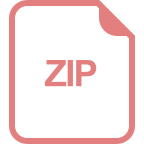
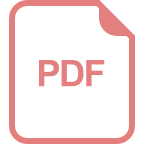
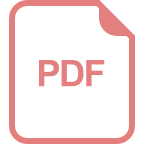
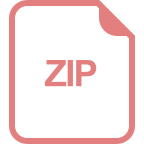
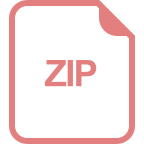
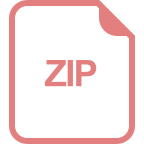