1、 货车要装载一批货物,货物由电视机、计算机和洗衣机3种商品组成。看出需要计算出整批货物的质量,具体要求如下: (1) 要求有一个ComputerWeight接口,该接口中有一个方法: public double computerWeight() (2) 要求有3个实现接口的类,即Television、Computer和WashMachine。这3个类通过实现接口computerTotalSales给出自重。 (3) 要求有一个Truck类,该类以ComputerWeight接口类型的数组作为成员(Truck类面向接口),那么该数组的单元可以存放Television对象的引用、Computer对象的引用或WashMachine对象的引用。程序能输出Truck对象所装载的货物的总质量。
时间: 2024-03-18 18:40:08 浏览: 23
好的,这是一个关于Java接口和类的问题。根据你的描述,可以先定义一个ComputerWeight接口,其中包含一个计算货物重量的方法computerWeight(),具体代码如下:
```java
public interface ComputerWeight {
double computerWeight();
}
```
然后,我们需要分别实现Television、Computer和WashMachine类,这三个类都需要实现ComputerWeight接口,并且给出自身的重量。具体代码如下:
```java
public class Television implements ComputerWeight {
private double weight = 20.0; // 电视机的重量为20kg
public double computerWeight() {
return weight;
}
}
public class Computer implements ComputerWeight {
private double weight = 10.0; // 计算机的重量为10kg
public double computerWeight() {
return weight;
}
}
public class WashMachine implements ComputerWeight {
private double weight = 30.0; // 洗衣机的重量为30kg
public double computerWeight() {
return weight;
}
}
```
最后,我们需要定义一个Truck类,该类以ComputerWeight接口类型的数组作为成员,可以存放Television对象的引用、Computer对象的引用或WashMachine对象的引用。程序能输出Truck对象所装载的货物的总质量。具体代码如下:
```java
public class Truck {
private ComputerWeight[] goods; // 存放货物的数组
public Truck(ComputerWeight[] goods) {
this.goods = goods;
}
public double getTotalWeight() {
double totalWeight = 0.0;
for (ComputerWeight good : goods) {
totalWeight += good.computerWeight();
}
return totalWeight;
}
}
```
在主函数中,我们可以先创建三种货物对象,并且将它们存放在ComputerWeight接口类型的数组中。然后,创建一个Truck对象,将这个数组作为构造函数的参数传入,最后调用Truck对象的getTotalWeight()方法,输出货车所装载的货物的总质量。具体代码如下:
```java
public static void main(String[] args) {
ComputerWeight[] goods = new ComputerWeight[3];
goods[0] = new Television();
goods[1] = new Computer();
goods[2] = new WashMachine();
Truck truck = new Truck(goods);
double totalWeight = truck.getTotalWeight();
System.out.println("货车所装载的货物的总质量为:" + totalWeight + "kg");
}
```
运行程序,输出的结果应该为:
```
货车所装载的货物的总质量为:60.0kg
```
相关推荐








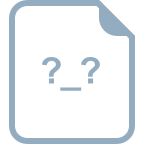